[Servlet/JSP] Lập trình Java Servlet cơ bản P6: Phiên làm việc (Session)
Trong bài viết này chúng ta cùng tìm hiểu phiên làm việc (session) là gì? Cách lấy thông tin của Session.
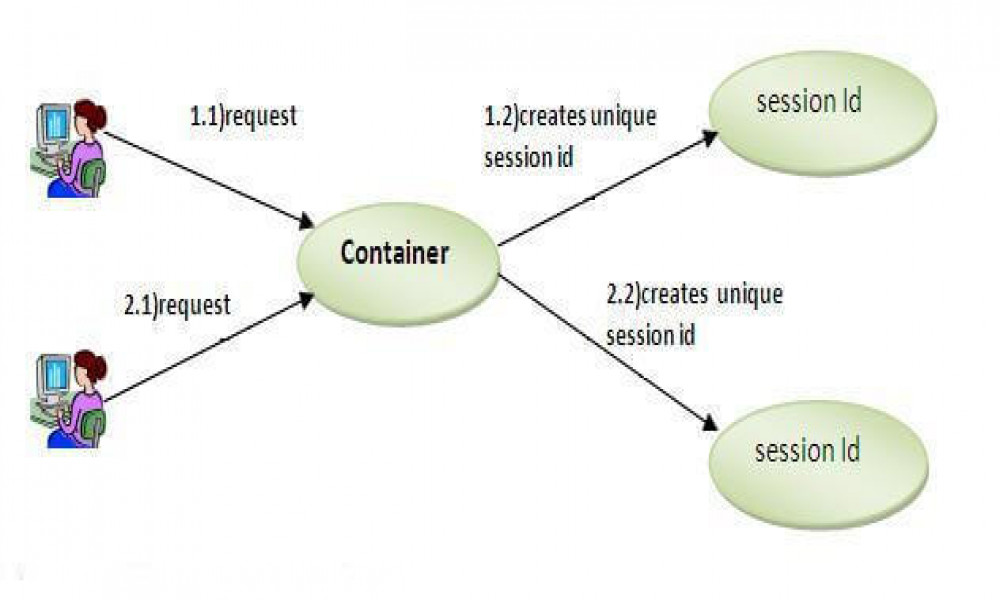
Để làm được hướng dẫn này, bạn cần thực hiện các hướng dẫn trong các bài viết trước:
3. Tạo và chạy Servlet đầu tiên
Xem bài viết trước tại đây
1. Phiên làm việc (Session)
Đối tượng HttpSession mô tả một phiên làm việc (session) của người dùng. Một phiên làm việc của người dùng chứa nhiều thông tin người dùng, và xuyên suốt trên các yêu cầu (request) đã gửi tới HTTP server.
Khi lần đầu tiên người dùng vào trang web của bạn, người dùng sẽ nhận được một ID duy nhất phân biệt với các người dùng khác. ID này thường được lưu trữ trong cookie hoặc tham số của request.
Đây là đoạn code để bạn truy cập vào đối tượng session:
protected void doGet(HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession();
}
Bạn có thể lưu trữ các giá trị vào đối tượng session, và lấy chúng ra sau đó, có thể là tại một trang khác. Trước hết, hãy xem cách bạn có thể lưu trữ giá trị vào trong đối tượng session:
// Lấy ra đối tượng HttpSession
HttpSession session = request.getSession();
// Giả sử người dùng đã login thành công.
UserInfo loginedInfo = new UserInfo("Tom", "USA", 5);
// Lưu trữ thông tin người dùng vào 1 thuộc tính (attribute) của Session.
// Bạn có thể lấy lại thông tin này bằng phương thức getAttribute.
session.setAttribute(Constants.SESSION_USER_KEY, loginedInfo);
Và lấy lại các thông tin đã lưu trữ trong Session tại một trang nào đó.
// Lấy ra đối tượng HttpSession.
HttpSession session = request.getSession();
// Lấy ra đối tượng UserInfo đã được lưu vào session
// sau khi người dùng login thành công.
UserInfo loginedInfo
= (UserInfo) session.getAttribute(Constants.SESSION_USER_KEY);
Xem ví dụ đầy đủ:
LoginServlet.java
package org.o7planning.tutorial.servlet.session;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.o7planning.tutorial.beans.Constants;
import org.o7planning.tutorial.beans.UserInfo;
@WebServlet(urlPatterns = { "/login" })
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public LoginServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
// Lấy ra đối tượng HttpSession
HttpSession session = request.getSession();
// Giả sử người dùng đã login thành công.
UserInfo loginedInfo = new UserInfo("Tom", "USA", 5);
// Lưu trữ thông tin người dùng vào 1 thuộc tính (attribute) của Session.
session.setAttribute(Constants.SESSION_USER_KEY, loginedInfo);
out.println("<html>");
out.println("<head><title>Session example</title></head>");
out.println("<body>");
out.println("<h3>You are logined!, info stored in session</h3>");
out.println("<a href='userInfo'>View User Info</a>");
out.println("</body>");
out.println("<html>");
}
}
UserInfoServlet.java
package org.o7planning.tutorial.servlet.session;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.o7planning.tutorial.beans.Constants;
import org.o7planning.tutorial.beans.UserInfo;
@WebServlet(urlPatterns = { "/userInfo" })
public class UserInfoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public UserInfoServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
// Lấy ra đối tượng HttpSession.
HttpSession session = request.getSession();
// Lấy ra đối tượng UserInfo đã được lưu vào session
// sau khi người dùng login thành công.
UserInfo loginedInfo = (UserInfo) session.getAttribute(Constants.SESSION_USER_KEY);
// Chưa login, Redirect (chuyển hướng) về trang login (LoginServlet).
if (loginedInfo == null) {
// ==> /ServletTutorial/login
response.sendRedirect(this.getServletContext().getContextPath() + "/login");
return;
}
out.println("<html>");
out.println("<head><title>Session example</title></head>");
out.println("<body>");
out.println("<h3>User Info:</h3>");
out.println("<p>User Name:" + loginedInfo.getUserName() + "</p>");
out.println("<p>Country:" + loginedInfo.getCountry() + "</p>");
out.println("<p>Post:" + loginedInfo.getPost() + "</p>");
out.println("</body>");
out.println("<html>");
}
}
Chạy ví dụ:
Xem bài viết tiếp theo tại đây.
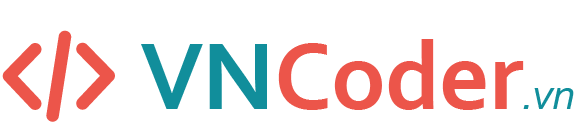
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết liên quan
Bài viết mới
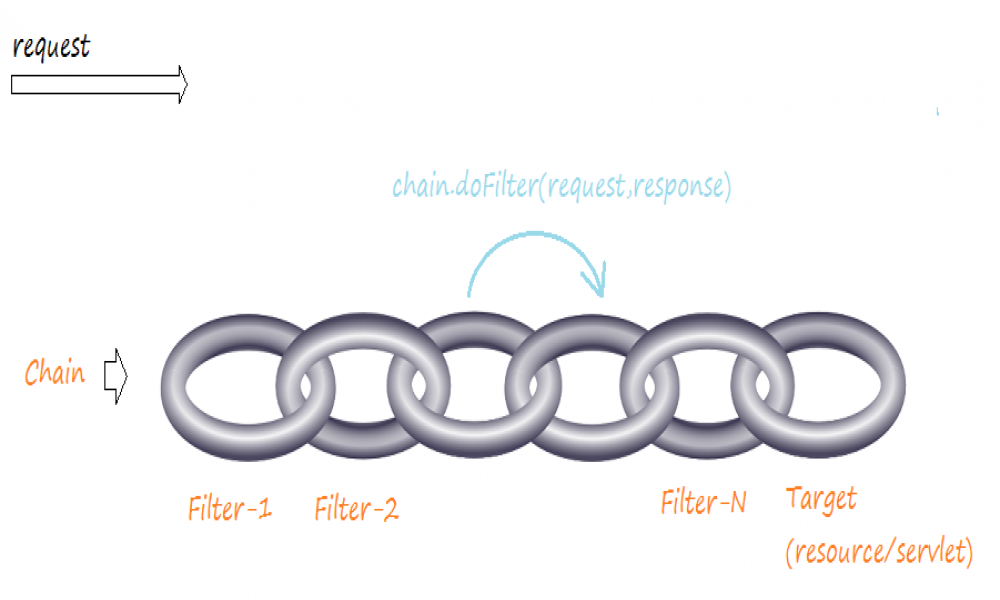
[Servlet/JSP] Lập trình Servlet Filter P3: Mô hình làm việc của Filter, Tham sô khởi tạo Servlet Filter
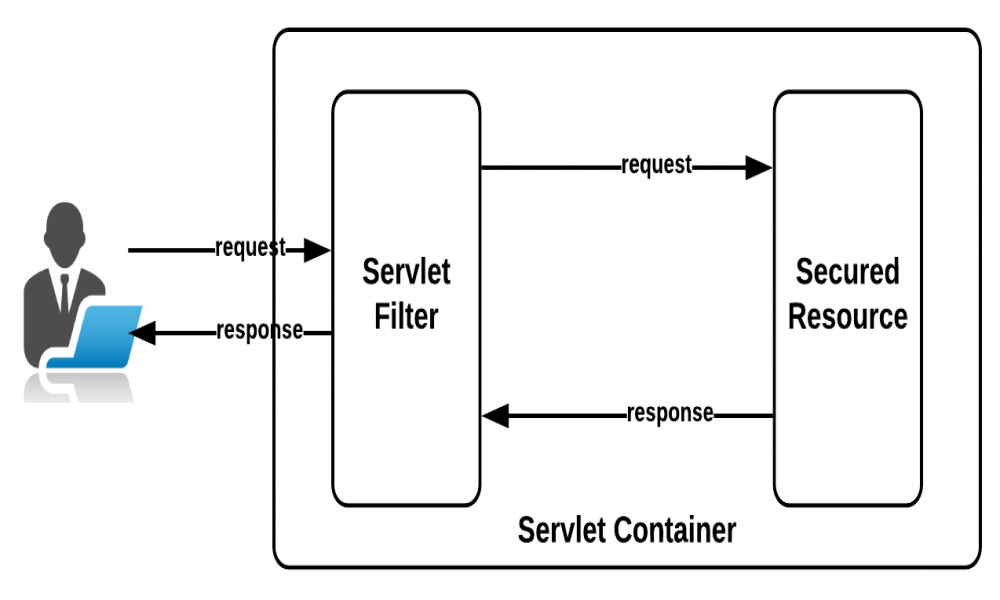
[Servlet/JSP] Lập trình Servlet Filter P2: Tạo Project Servlet Filter, cấu hình môi trường chạy Servlet Filter.
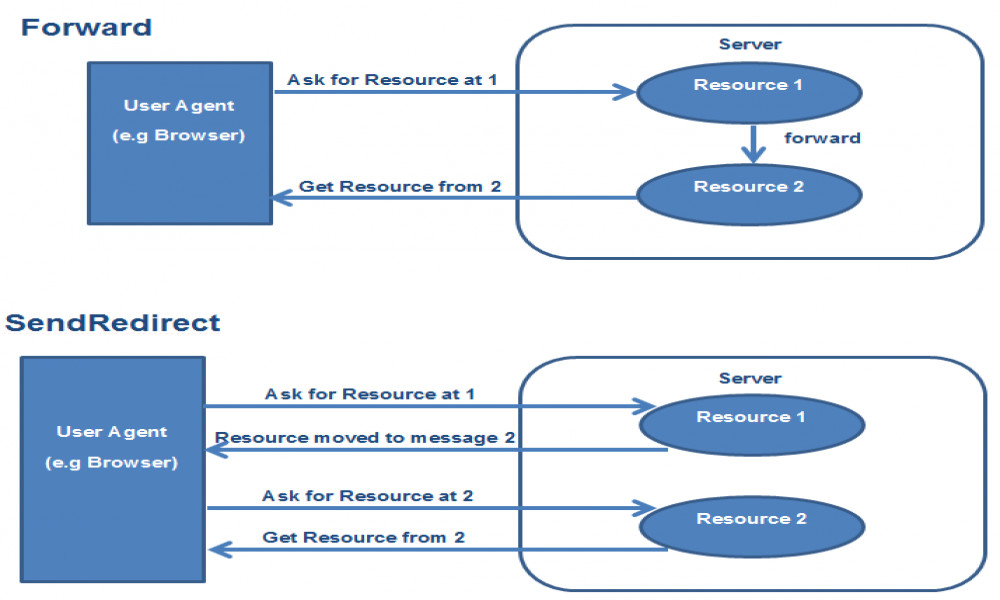