API Authentication sử dụng JWT trong Laravel
Hôm nay mình xin giới thiệu tới các bạn một ví dụ về API Authentication trong Laravel sử dụng JWT. Đây là một ví dụ mà mình thấy khá hữu ích trong việc xác thực người dùng trong các ứng dụng web.
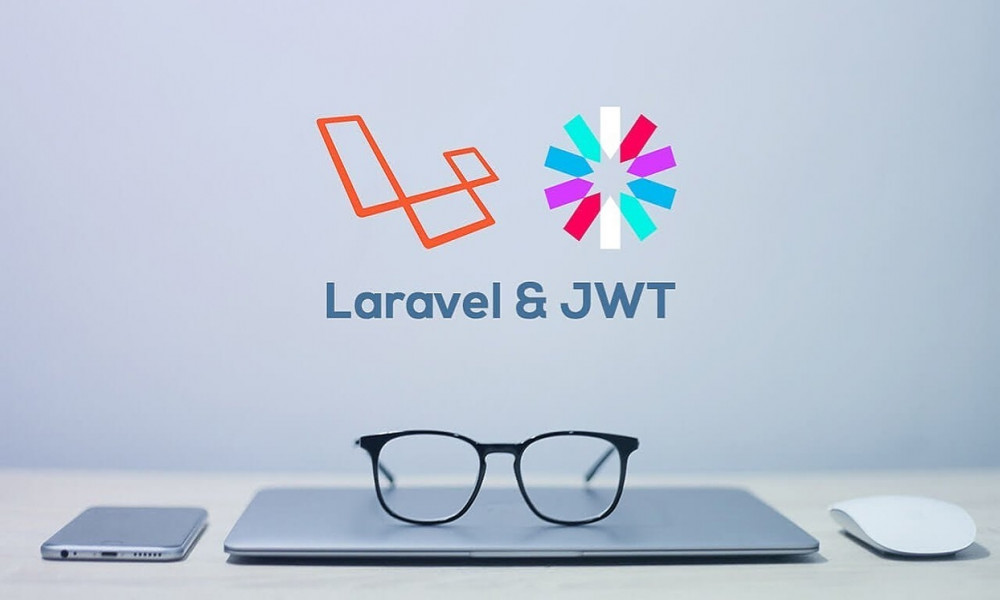
1. Giới thiệu
Hôm nay mình xin giới thiệu tới các bạn một ví dụ về API Authentication trong Laravel sử dụng JWT. Đây là một ví dụ mà mình thấy khá hữu ích trong việc xác thực người dùng trong các ứng dụng web.
JSON Web Token (JWT) là một tiêu chuẩn mở (RFC 7519) định nghĩa một cách nhỏ gọn và an toàn để truyền tải thông tin giữa các bên một cách an toàn dưới dạng 1 đối tượng JSON . Các thông tin này được xác thực và có độ tin cậy cao vì nó có chứa chữ ký số (digital signature). Để hiểu rõ hơn về JWT mời bạn đọc bài viết JSON Web Token (JWT) là gì?
2. Cài đặt package JWT
Thêm package tymon/jwt-auth vào file composer.json:
composer require tymon/jwt-auth "1.0.*"
Để mã hóa token, chúng ta cần tạo secret key:
php artisan jwt:generate
Tiến hành publish file config JWT. Khi publish thành công, ta sẽ thấy file config/jwt.php được tạo mới. Để publish file config trong Laravel, chạy command line sau đây:
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
Mở file /app/Http/Kernel.php và thêm 2 dòng vào cuối của $routeMiddleware:
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'bindings' => \Illuminate\Routing\Middleware\SubstituteBindings::class,
'cache.headers' => \Illuminate\Http\Middleware\SetCacheHeaders::class,
'can' => \Illuminate\Auth\Middleware\Authorize::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
'signed' => \Illuminate\Routing\Middleware\ValidateSignature::class,
'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class,
'verified' => \Illuminate\Auth\Middleware\EnsureEmailIsVerified::class,
// THIS LINES HAS BEEN ADDED to JWT Implementation
'jwt.auth' => \Tymon\JWTAuth\Http\Middleware\Authenticate::class,
'jwt.refresh' => \Tymon\JWTAuth\Http\Middleware\RefreshToken::class,
];
Mở file /app/User.php và impliments class JWTSubject :
<?php
namespace App;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
// Please add this line
use Tymon\JWTAuth\Contracts\JWTSubject;
class User extends Authenticatable implements JWTSubject
{
// BODY OF THIS CLASS
public function getJWTIdentifier()
{
return $this->getKey();
}
public function getJWTCustomClaims()
{
return [];
}
}
Mặc định api authentication sử dụng token, ta sửa lại như sau:
<?php
// OTHER ARRAYS
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'jwt',
'provider' => 'users',
],
],
Quá trình cài đặt đã xong, để kiểm tra JWT đã chạy được chưa ta tạo controller /app/Http/Controllers/APILoginController.php để thực hiện kiểm tra chức năng login:
<?php
namespace app\Http\Controllers;
use Illuminate\Http\Request;
class APILoginController extends Controller
{
public function login()
{
// get email and password from request
$credentials = request(['email', 'password']);
// try to auth and get the token using api authentication
if (!$token = auth('api')->attempt($credentials)) {
// if the credentials are wrong we send an unauthorized error in json format
return response()->json(['error' => 'Unauthorized'], 401);
}
return response()->json([
'token' => $token,
'type' => 'bearer', // you can ommit this
'expires' => auth('api')->factory()->getTTL() * 60, // time to expiration
]);
}
}
Tạo route để login và lấy token được trả về
Route::post('login', 'APILoginController@login');
Sử dụng postman để gửi request, khi đăng nhập thành công kết quả trả về có chứa token:
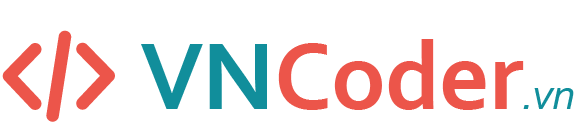
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết mới
Được xem nhiều nhất
[Laravel] Hướng dẫn tích hợp thanh toán online, tích hợp cổng thanh to...
[Laravel] Sử dụng Ajax làm chức năng tìm kiếm trong Laravel
[Laravel] Cách sử dụng Charts - hướng dẫn vẽ biểu đồ trong Laravel
[Laravel] Hướng dẫn sử dụng Raw DB Query trong Laravel
[Laravel] Hướng dẫn đăng nhập, đăng ký tài khoản bằng tài khoản Facebo...
Khóa học liên quan
Xây dựng ứng dụng với Laravel và Vuejs
Lượt xem: 16287
Chuyên mục: Laravel