[Laravel] Sử dụng Ajax làm chức năng tìm kiếm trong Laravel
Chức năng tìm kiếm sử dụng Ajax được rất nhiều các trang Web sử dụng, việc sử dụng ajax cho chức năng tìm kiếm rất tiện lợi - giúp kết quả tìm kiếm được hiển thị mà không cần load lại trang Web. Vì vậy trong bài viết này vncoder.vn sẽ hướng dẫn các bạn làm chức năng tìm kiếm sử dụng Ajax trong Laravel.
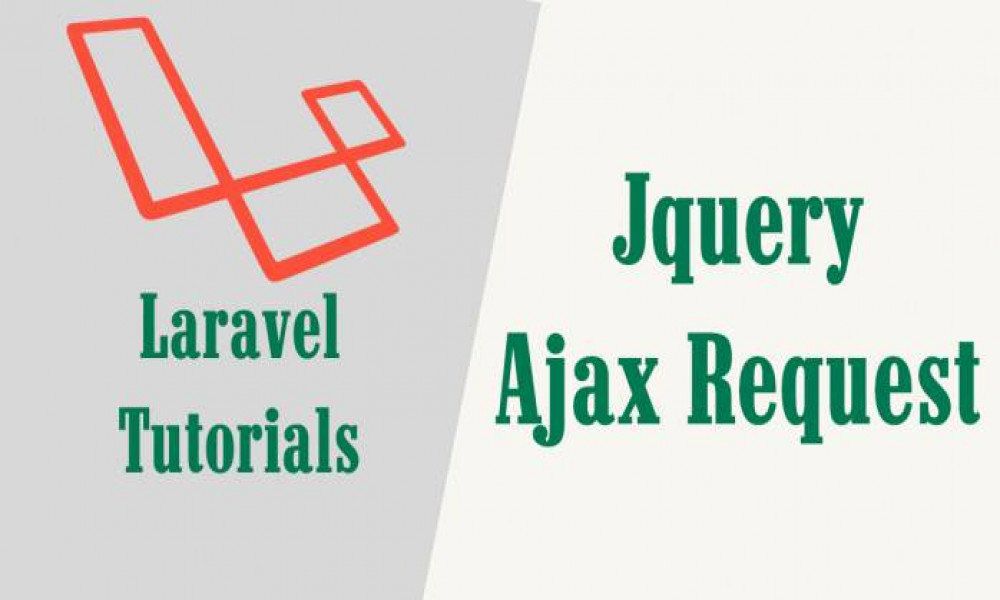
Chức năng tìm kiếm sử dụng Ajax được rất nhiều các trang Web sử dụng, việc sử dụng ajax cho chức năng tìm kiếm rất tiện lợi - giúp kết quả tìm kiếm được hiển thị mà không cần load lại trang Web. Vì vậy trong bài viết này vncoder.vn sẽ hướng dẫn các bạn làm chức năng tìm kiếm sử dụng Ajax trong Laravel.
Tạo bảng dữ liệu để tìm kiếm.
Tạo bảng với migrate.
Tất nhiên rồi, tìm kiếm thì cần có bảng dữ liệu để tìm kiếm. Chúng ta tạo bảng có tên là products
php artisan make:migration products --create=products
Các bạn vào database và mở file database/migrations/...products.php lên và chèn đoạn code sau:
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class Products extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->increments('id');
$table->string('name_product');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('products');
}
}
Chúng ta tạo trường 'name_product' để tìm kiếm với nó các bạn thích tìm kiếm với trường nào thì thêm vào và có thể thêm tùy ý miễn là chú ý khi truy vấn dữ liệu để không bị nhầm tên là được. Bây giờ các bạn tạo bảng với câu lệnh.
php artisan migrate
Chú ý là để chạy migrate nhớ cấu hình cho file .env và tạo tên database tương ứng nhé
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=shop
DB_USERNAME=root
DB_PASSWORD=
Tên database là 'shop' còn các bạn có thể tạo database khác và kết nối cho đúng là được.
Tạo dữ liệu mẫu với seeder
Để tạo dữ liệu mẫu chúng ta có thể tạo trực tiếp trong cơ sở dữ liệu hoặc sử dung seeder. Câu lệnh tạo seeder.
php artisan make:seeder ProductTableSeeder
Vào seeds mở file vừa tạo lên để thêm dữ liệu mẫu vào.
<?php
use Illuminate\Database\Seeder;
class ProductTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
//
$data = [
[
'name_product'=>'iPhone',
],
[
'name_product'=>'Samsung',
],
[
'name_product'=>'HTC',
],
[
'name_product'=>'Huawei',
],
[
'name_product'=>'Oppo',
],
];
DB::table('products')->insert($data);
}
}
Giờ chạy seeder nào.
php artisan db:seed --class=ProductTableSeeder
Tạo routes.
Vào web.php thêm hai routes như sau.
Route::get('search', 'SearchController@getSearch');
Route::post('search/name', 'SearchController@getSearchAjax')->name('search');
Tạo Controller.
Có routes rồi thì phải tạo controller để chạy chứ đúng không nào. Lệnh tạo controller, ở đây chúng ta tạo controller có tên là 'SearchController' các bạn có thể đặt tên khác tùy thích nhưng phải trùng với route nhé.
php artisan make:controller SearchController
Tạo view.
Vào view và tạo một view với tên là 'searchajax.blade.php' và tạo dao diện search như sau.
<!DOCTYPE html>
<html>
<head>
<title>Ajax search</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<style type="text/css">
.box{
width:600px;
margin:0 auto;
}
</style>
</head>
<body>
<br />
<div class="container box">
<h3 align="center">Gợi ý tìm kiếm với ajax</h3><br />
<div class="form-group">
<input type="text" name="country_name" id="country_name" class="form-control input-lg" placeholder="Enter Country Name" />
<div id="countryList"><br>
</div>
</div>
{{ csrf_field() }}
</div>
</body>
</html>
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class SearchController extends Controller
{
public function getSearch(Request $request)
{
return view('searchajax');
}
}
Chúng ta bắt đầu chạy thử xem đã hiển thị được view searchajax.blade.php chưa.
Gợi ý tìm kiếm với ajax.
Bây giờ mới đến phần chính. Trong view thêm đoạn code ajax để tìm kiếm và hiển thị như sau.
<!DOCTYPE html>
<html>
<head>
<title>Ajax search</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<style type="text/css">
.box{
width:600px;
margin:0 auto;
}
</style>
</head>
<body>
<br />
<div class="container box">
<h3 align="center">Gợi ý tìm kiếm với ajax</h3><br />
<div class="form-group">
<input type="text" name="country_name" id="country_name" class="form-control input-lg" placeholder="Enter Country Name" />
<div id="countryList"><br>
</div>
</div>
{{ csrf_field() }}
</div>
</body>
</html>
<script>
$(document).ready(function(){
$('#country_name').keyup(function(){ //bắt sự kiện keyup khi người dùng gõ từ khóa tim kiếm
var query = $(this).val(); //lấy gía trị ng dùng gõ
if(query != '') //kiểm tra khác rỗng thì thực hiện đoạn lệnh bên dưới
{
var _token = $('input[name="_token"]').val(); // token để mã hóa dữ liệu
$.ajax({
url:"{{ route('search') }}", // đường dẫn khi gửi dữ liệu đi 'search' là tên route mình đặt bạn mở route lên xem là hiểu nó là cái j.
method:"POST", // phương thức gửi dữ liệu.
data:{query:query, _token:_token},
success:function(data){ //dữ liệu nhận về
$('#countryList').fadeIn();
$('#countryList').html(data); //nhận dữ liệu dạng html và gán vào cặp thẻ có id là countryList
}
});
}
});
$(document).on('click', 'li', function(){
$('#country_name').val($(this).text());
$('#countryList').fadeOut();
});
});
</script>
Bây giờ bên controller sẽ sử lý khi nhận được dữ liệu.
<?php
namespace App\Http\Controllers;
use DB;
use Illuminate\Http\Request;
class SearchController extends Controller
{
public function getSearch(Request $request)
{
return view('searchajax');
}
function getSearchAjax(Request $request)
{
if($request->get('query'))
{
$query = $request->get('query');
$data = DB::table('products')
->where('name_product', 'LIKE', "%{$query}%")
->get();
$output = '<ul class="dropdown-menu" style="display:block; position:relative">';
foreach($data as $row)
{
$output .= '
<li><a href="data/'. $row->id .'">'.$row->name_product.'</a></li>
';
}
$output .= '</ul>';
echo $output;
}
}
}
vậy là ok rồi đấy.
Lưu ý:
Có thể chỉnh sửa hiển thị tùy ý bằng cấu hình trong controller như thêm đường dẫn, hiển thị thêm ảnh, tên, ... Thậm chí còn css được, ví dụ:
$output = '<ul class="dropdown-menu" style="display:block; position:relative">';
foreach($data as $row)
{
$output .= '
<li><a href="data/'. $row->id .'">'.$row->name_product.'</a></li>
<img src="public/'.$row->img.'"></img>
<p style="color:red; text-align:center">abc</p>
';
}
$output .= '</ul>';
Bạn có thể trả về dữ liệu kiểu json và đọc json đấy bên code của ajax bằng cách trả về kiểu dữ liệu json thay vì kiểu html vd: return response()->json($products);
Kết luận
Vậy là vncoder.vn đã hướng dẫn xong gợi ý tìm kiếm với ajax trong Laravel, hy vọng các bạn đọc và làm theo thành công. Hãy để lại bình luận nếu bài viết có ích cho bạn. Chúc các bạn thành công!.
Tài liệu tham khảo
https://viblo.asia/p/goi-y-tim-kiem-voi-ajax-trong-laravel-XL6lAxVrZek
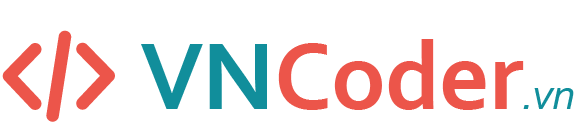
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết mới
Được xem nhiều nhất
[Laravel] Hướng dẫn tích hợp thanh toán online, tích hợp cổng thanh to...
[Laravel] Sử dụng Ajax làm chức năng tìm kiếm trong Laravel
[Laravel] Cách sử dụng Charts - hướng dẫn vẽ biểu đồ trong Laravel
[Laravel] Hướng dẫn sử dụng Raw DB Query trong Laravel
[Laravel] Hướng dẫn đăng nhập, đăng ký tài khoản bằng tài khoản Facebo...
Khóa học liên quan
Xây dựng ứng dụng với Laravel và Vuejs
Lượt xem: 16337
Chuyên mục: Laravel