- Bài 1: Tổng quan ngôn ngữ Java
- Bài 2: Hướng dẫn cài đặt và cấu hình Java
- Bài 3: Hướng dẫn cài đặt và cấu hình Intellij IDEA trên Window
- Bài 4: Tạo Project và làm quen với Intelij IDEA
- Bài 5: Cú pháp và quy tắc cơ bản trong Java
- Bài 6: Các kiểu dữ liệu trong Java
- Bài 7: Các kiểu biến trong Java
- Bài 8: Toán tử trong Java
- Bài 9: Hệ thống Unicode trong Java
- Bài 10: Các kiểu vòng lặp
- Bài 11: Câu lệnh điều khiển rẽ nhánh
- Bài 12: Câu lệnh Break, Continue trong Java
- Bài 13: Lớp và đối tượng
- Bài 14: Tính chất của lập trình hướng đối tượng trong Java
- Bài 15: Abstract class và Interface trong Java
- Bài 16: Từ khóa super và this trong Java
- Bài 17: Từ khóa static và final trong Java
- Bài 18: Mảng (Array) trong Java
- Bài 19: Package trong Java
- Bài 21: String trong Java
- Bài 22: StringBuffer và StringBuilder
- Bài 23: Tổng quan về File và I/O
- Bài 24: Xử lý nhập xuất bằng Byte Stream và Character Stream
- Bài 25: Thao tác với tệp và thư mục trong Java
- Bài 26: Tổng quan về Collection trong Java
- Bài 27: Những Interface phổ biến trong Java Collection Framework
- Bài 28: Những Class được triển khai dựa trên Interface của Java Collection
- Bài 29: Khái niệm xử lý ngoại lệ trong Java
- Bài 30: Hướng dẫn xử lý ngoại lệ trong Java
- Bài 31: Đa luồng trong Java
- Bài 32: Thread synchronization trong Java
- Bài 33: Tổng quan về Java AWT
- Bài 34: Container trong Java AWT
- Bài 35: Component trong Java AWT
- Bài 36: Layout Manager trong Java AWT
- Bài 37: Xử lý sự kiện trong Java AWT
- Bài 38: Tổng quan về Java Swing
- Bài 39: Container trong Java Swing
- Bài 40: Component trong Java Swing
- Bài 41: Layout trong Java Swing
- Bài 42: Event trong Java Swing
- Bài 43: Menu trong Java Swing
- Bài 44: Kết nối cơ sở dữ liệu với thư viện JDBC trong Java
Bài 37: Xử lý sự kiện trong Java AWT - Lập trình Java cơ bản
Đăng bởi: Admin | Lượt xem: 5273 | Chuyên mục: Java
Trạng thái của một đối tượng bị thay đổi được gọi là một sự kiện (event). Ví dụ, bấm vào nút, kéo chuột, vv Gói java.awt.event cung cấp nhiều lớp sự kiện và interface Listener để xử lý sự kiện Java AWT.
1. Các lớp Java Event và các interface Listener
Các lớp Event | Các interface Listener |
---|---|
ActionEvent | ActionListener |
MouseEvent | MouseListener and MouseMotionListener |
MouseWheelEvent | MouseWheelListener |
KeyEvent | KeyListener |
ItemEvent | ItemListener |
TextEvent | TextListener |
AdjustmentEvent | AdjustmentListener |
WindowEvent | WindowListener |
ComponentEvent | ComponentListener |
ContainerEvent | ContainerListener |
FocusEvent | FocusListener |
Phương thức đăng ký
Để đăng ký thành phần với trình Listener, có nhiều lớp cung cấp các phương thức đăng ký. Ví dụ:
- Buttonpublic void addActionListener(ActionListener a){}
- MenuItempublic void addActionListener(ActionListener a){}
- TextFieldpublic void addActionListener(ActionListener a){}public void addTextListener(TextListener a){}
- TextAreapublic void addTextListener(TextListener a){}
- Checkboxpublic void addItemListener(ItemListener a){}
- Choicepublic void addItemListener(ItemListener a){}
- Listpublic void addActionListener(ActionListener a){}public void addItemListener(ItemListener a){}
2. Ví dụ xử lý sự kiện trong Java AWT
Chúng tôi có thể đặt mã xử lý sự kiện vào một trong các vị trí sau:
- Bên trong lớp hiện tại.
- Bên trong lớp khác.
- Bên trong lớp nặc danh.
Xử lý sự kiện Java AWT - bên trong lớp hiện tại
import java.awt.Button;
import java.awt.Frame;
import java.awt.TextField;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class AWTEventHandling1 extends Frame implements ActionListener {
private TextField textField;
public AWTEventHandling1() {
// tạo các thành phần
textField = new TextField();
textField.setBounds(60, 80, 170, 20);
Button button = new Button("click me");
button.setBounds(100, 120, 80, 30);
// đăng ký trình listener
button.addActionListener(this);
// thêm thành phần, kích thước, layout, khả năng hiển thị
setTitle("Vi du xu ly su kien Java AWT");
add(button);
add(textField);
setSize(400, 300);
setLayout(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
textField.setText("Welcome to Java AWT");
}
public static void main(String args[]) {
new AWTEventHandling1();
}
}
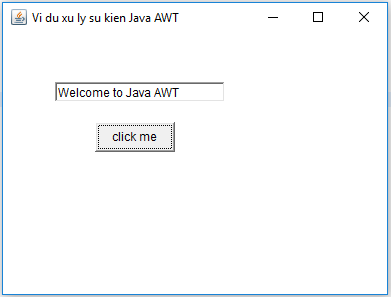
Xử lý sự kiện Java AWT - bên trong lớp hiện khác
Tạo lớp view: AWTView.java:
import java.awt.Button;
import java.awt.Frame;
import java.awt.TextField;
public class AWTView extends Frame {
TextField textField;
AWTView() {
// tạo các thành phần
textField = new TextField();
textField.setBounds(60, 80, 170, 20);
Button button = new Button("click me");
button.setBounds(100, 120, 80, 30);
// đăng ký listener
Controller obj = new Controller(this);
button.addActionListener(obj);
// thêm thành phần, kích thước, layout, khả năng hiển thị
setTitle("Vi du xu ly su kien Java AWT");
add(button);
add(textField);
setSize(400, 300);
setLayout(null);
setVisible(true);
}
}
Tạo lớp controller: Controller.java:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Controller implements ActionListener {
AWTView obj;
Controller(AWTView obj) {
this.obj = obj;
}
@Override
public void actionPerformed(ActionEvent e) {
obj.textField.setText("Welcome to Java AWT");
}
}
Tạo lớp AWTEventHandling2.java:
public class AWTEventHandling2 {
public static void main(String[] args) {
AWTView view = new AWTView();
}
}
Kết quả:
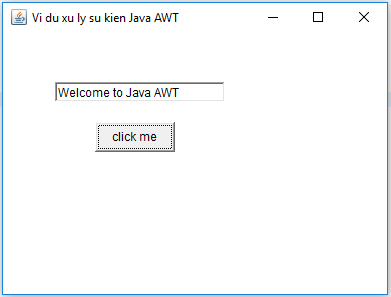
Xử lý sự kiện Java AWT - bên trong lớp nặc danh
import java.awt.Button;
import java.awt.Frame;
import java.awt.TextField;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class AWTEventHandling3 extends Frame {
TextField textField;
AWTEventHandling3() {
textField = new TextField();
textField.setBounds(60, 50, 170, 20);
Button button = new Button("click me");
button.setBounds(50, 120, 80, 30);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
textField.setText("Hello World!");
}
});
setTitle("Vi du xu ly su kien Java AWT");
add(button);
add(textField);
setSize(400, 300);
setLayout(null);
setVisible(true);
}
public static void main(String args[]) {
new AWTEventHandling3();
}
}
Kết quả:
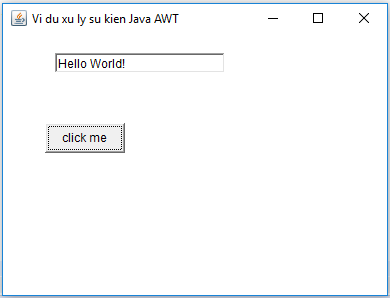
Như vậy, thông qua bài học này, mình đã giới thiệu đến các bạn các phương thức xử lý sự kiện trong Javá AWT. Cảm ơn các bạn đã đọc. Sang bài tiếp theo, chúng ta sẽ cùng tìm hiểu một thư viện hỗ trợ xây dựng giao diện khác cải tiến hơn so với Java AWT, đó là Java Swing.
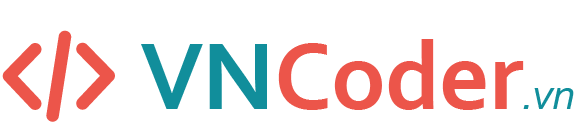
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
- Bài 1: Tổng quan ngôn ngữ Java
- Bài 2: Hướng dẫn cài đặt và cấu hình Java
- Bài 3: Hướng dẫn cài đặt và cấu hình Intellij IDEA trên Window
- Bài 4: Tạo Project và làm quen với Intelij IDEA
- Bài 5: Cú pháp và quy tắc cơ bản trong Java
- Bài 6: Các kiểu dữ liệu trong Java
- Bài 7: Các kiểu biến trong Java
- Bài 8: Toán tử trong Java
- Bài 9: Hệ thống Unicode trong Java
- Bài 10: Các kiểu vòng lặp
- Bài 11: Câu lệnh điều khiển rẽ nhánh
- Bài 12: Câu lệnh Break, Continue trong Java
- Bài 13: Lớp và đối tượng
- Bài 14: Tính chất của lập trình hướng đối tượng trong Java
- Bài 15: Abstract class và Interface trong Java
- Bài 16: Từ khóa super và this trong Java
- Bài 17: Từ khóa static và final trong Java
- Bài 18: Mảng (Array) trong Java
- Bài 19: Package trong Java
- Bài 21: String trong Java
- Bài 22: StringBuffer và StringBuilder
- Bài 23: Tổng quan về File và I/O
- Bài 24: Xử lý nhập xuất bằng Byte Stream và Character Stream
- Bài 25: Thao tác với tệp và thư mục trong Java
- Bài 26: Tổng quan về Collection trong Java
- Bài 27: Những Interface phổ biến trong Java Collection Framework
- Bài 28: Những Class được triển khai dựa trên Interface của Java Collection
- Bài 29: Khái niệm xử lý ngoại lệ trong Java
- Bài 30: Hướng dẫn xử lý ngoại lệ trong Java
- Bài 31: Đa luồng trong Java
- Bài 32: Thread synchronization trong Java
- Bài 33: Tổng quan về Java AWT
- Bài 34: Container trong Java AWT
- Bài 35: Component trong Java AWT
- Bài 36: Layout Manager trong Java AWT
- Bài 37: Xử lý sự kiện trong Java AWT
- Bài 38: Tổng quan về Java Swing
- Bài 39: Container trong Java Swing
- Bài 40: Component trong Java Swing
- Bài 41: Layout trong Java Swing
- Bài 42: Event trong Java Swing
- Bài 43: Menu trong Java Swing
- Bài 44: Kết nối cơ sở dữ liệu với thư viện JDBC trong Java