CÁCH TẠO ỨNG DỤNG TRÌNH XEM PDF TRÊN ANDROID
Khi bạn truy cập Cửa hàng Google Play, có rất nhiều ứng dụng bạn có thể tải xuống và sử dụng để mở hoặc xem tệp pdf trong thiết bị của mình. Nếu bạn đang tự hỏi làm thế nào ứng dụng này được tạo ra, đừng lo lắng vì bạn sẽ có các công cụ và kiến thức bạn cần ở hướng dẫn này.
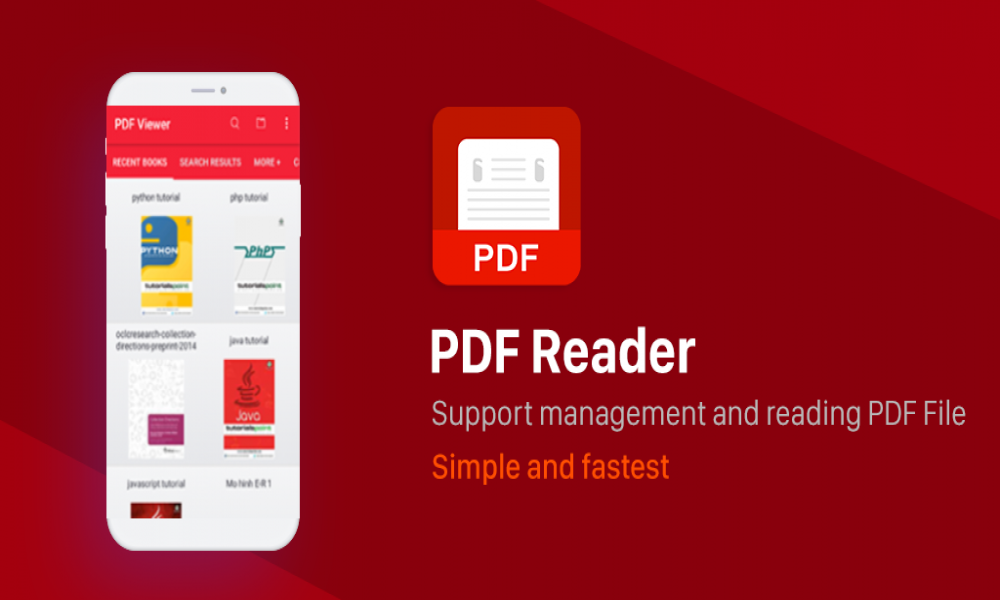
Dưới đây là ảnh chụp màn hình về những gì chúng ta sẽ tạo
1. TẠO MỘT DỰ ÁN ANDROID MỚI
- Mở Android Studio
- Chuyển đến file menu
- Chọn new
- Nhập project name
- Nhập activity name
- Giữ các cài đặt mặc định khác
- Click vào nút finish để tạo một dự án Android mới
2. LIỆT KÊ TẤT CẢ CÁC TỆP PDF TRONG THIẾT BỊ
Có nhiều cách khác nhau mà chúng ta có thể sử dụng để truy cập danh sách tệp PDF trong thiết bị của mình: chúng ta có thể sử dụng Ứng dụng Trình quản lý tệp hoặc Công cụ chọn tệp hoặc chúng ta có thể tự sử dụng Android API.
Trong hướng dẫn này chúng ta sử dụng Android API.
public class ListPDFUtils {
private static final String TAG = ListPDFUtils.class.getSimpleName();
private Context context;
public ListPDFUtils(Context context) {
this.context = context;
}
String[] projection = {
MediaStore.Files.FileColumns._ID,
MediaStore.Files.FileColumns.MIME_TYPE,
MediaStore.Files.FileColumns.DATE_ADDED,
MediaStore.Files.FileColumns.DATE_MODIFIED,
MediaStore.Files.FileColumns.DISPLAY_NAME,
MediaStore.Files.FileColumns.TITLE,
MediaStore.Files.FileColumns.SIZE,
};
public List<Document> queryMediaStore(String fileMimeType){
String whereClause = getFileType(fileMimeType);
String orderBy = MediaStore.Files.FileColumns.SIZE + " DESC";
Uri uri = MediaStore.Files.getContentUri("external");
Cursor cursor = context.getContentResolver().query(uri, projection, whereClause, null, orderBy);
int idCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns._ID);
int mimeCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.MIME_TYPE);
int addedCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.DATE_ADDED);
int modifiedCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.DATE_MODIFIED);
int nameCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.DISPLAY_NAME);
int titleCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.TITLE);
int sizeCol = cursor.getColumnIndexOrThrow(MediaStore.Files.FileColumns.SIZE);
List<Document> allDoc = new ArrayList<>();
if (cursor.moveToFirst()) {
do {
Uri fileUri = Uri.withAppendedPath(uri, cursor.getString(idCol));
String mimeType = cursor.getString(mimeCol);
long dateAdded = cursor.getLong(addedCol);
long dateModified = cursor.getLong(modifiedCol);
String name = cursor.getString(nameCol);
String title = cursor.getString(titleCol);
long size = cursor.getLong(sizeCol);
String path =getRealPathFromURI(context, fileUri);
String extractName = StringUtils.capitalize(FilenameUtils.getName(path));
Log.d(TAG, "File in PDF " + title);
Document document = new Document(extractName, Utils.formatSize(size), Utils.formatDate(dateModified), path);
int icon = getIcon(fileMimeType);
document.setIcon(icon);
allDoc.add(document);
} while (cursor.moveToNext());
}
cursor.close();
return allDoc;
}
public String getRealPathFromURI(Context context, Uri contentUri) {
Cursor cursor = null;
try {
String[] proj = { MediaStore.Images.Media.DATA };
cursor = context.getContentResolver().query(contentUri, proj, null, null, null);
int column_index = cursor.getColumnIndexOrThrow(MediaStore.Images.Media.DATA);
cursor.moveToFirst();
return cursor.getString(column_index);
} finally {
if (cursor != null) {
cursor.close();
}
}
}
}
3. THÊM PERMISSION
Vì việc truy cập Android storage API cần có quyền, chúng ta sẽ thêm mã này vào tệp manifest.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
4. THÊM THƯ VIỆN BÊN THỨ BA CỦA ANDROID
Chúng ta sẽ thêm một số thư viện android sẽ giúp chúng ta giải quyết nhanh một số vấn đề.
Mở tệp build.gradle và thêm các thư viện bên dưới.
implementation 'com.github.burakeregar:EasiestGenericRecyclerAdapter:v1.5'
implementation 'com.github.barteksc:android-pdf-viewer:3.2.0-beta.1'
Chúng ta sẽ sử dụng EasiestGenericRecyclerAdapter
để hiển thị tất cả các tệp PDF mà chúng ta đã tìm nạp từ thiết bị của mình.
Chúng ta sẽ sử dụng trình xem PDF để xem nội dung của từng tệp PDF.
5. LIỆT KÊ TỆP PDF VỚI EASHESTGENERICRECYCLER ADAPTER
private void populateRecyclerView() {
Observable<List<Document>> pdfs = Observable.fromCallable(new Callable<List<Document>>() {
@Override
public List<Document> call() throws Exception {
//return loadPdf();
return listPDFUtils.queryMediaStore(ListPDFUtils.FileMimeTypes.PDF_MIME_TYPE);
}
});
compositeDisposable.add(pdfs.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Consumer<List<Document>>() {
@Override
public void accept(List<Document> documents) throws Exception {
progressBar.setVisibility(View.GONE);
recyclerView.setVisibility(View.VISIBLE);
setUpUIList(documents);
}
})
);
}
private void setUpUIList(List<Document> data){
adapter = new GenericAdapterBuilder()
.addModel(R.layout.list_pdf_item_layout, PdfViewHolder.class, Document.class)
.setFilterEnabled()
.execute();
recyclerView.setAdapter(adapter);
adapter.setList(data);
adapter.setFilter(new SearchFilter(adapter));
}
6. TẠO MỘT ACTIVITY MỚI
Bây giờ chúng ta sẽ tạo một activity mới mà chúng ta sẽ sử dụng để hiển thị một tệp PDF duy nhất.
Mở fie layout của activity mới.
<com.github.barteksc.pdfviewer.PDFView
android:id="@+id/pdf_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginBottom="8dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toTopOf="@id/edit_box"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"/>
Chúng ta sẽ lấy tham chiếu PDFView trong activity. Khi một tệp PDF được click vào, chúng ta sẽ nhận được các thuộc tính File được bao bọc trong một lớp Document.
Dưới đây là code để xem tệp PDF.
public PDFView.Configurator getPdfConfigurator(PDFView pdfView){
PDFView.Configurator configurator = null;
if (doc != null) {
configurator = pdfView.fromUri(getFileUri(context, doc.getDocumentPath()))
.enableDoubletap(true)
.enableSwipe(true)
.swipeHorizontal(true)
.enableAnnotationRendering(true)
.pageFitPolicy(FitPolicy.BOTH)
.fitEachPage(true)
.spacing(2);
}
return configurator;
}
PDFView.Configurator configurator = getPdfConfigurator(pdfView);
configurator.onPageChange(new OnPageChangeListener() {
@Override
public void onPageChanged(int page, int pageCount) {
currentIndexPage = page;
setPDFPaging(page, pageCount);
}
}).onTap(new OnTapListener() {
@Override
public boolean onTap(MotionEvent e) {
return false;
}
}).load();
Bạn có thể xem bất kỳ tệp PDF nào trong thiết bị của mình.
Nếu bạn có câu hỏi liên quan đến hướng dẫn này, vui lòng để lại bình luận bên dưới.
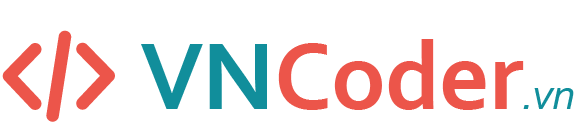
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết liên quan
Bài viết mới
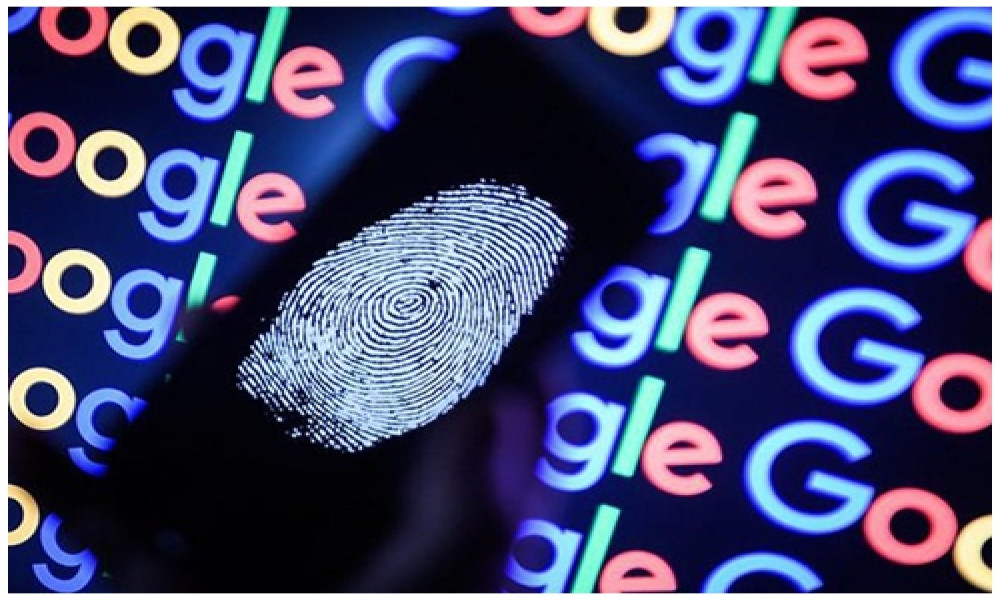
Hướng dẫn xây dựng ứng dụng sử dụng API vân tay (FINGERPRINT API) để đăng nhập, đăng ký người dùng trong Android (P2)
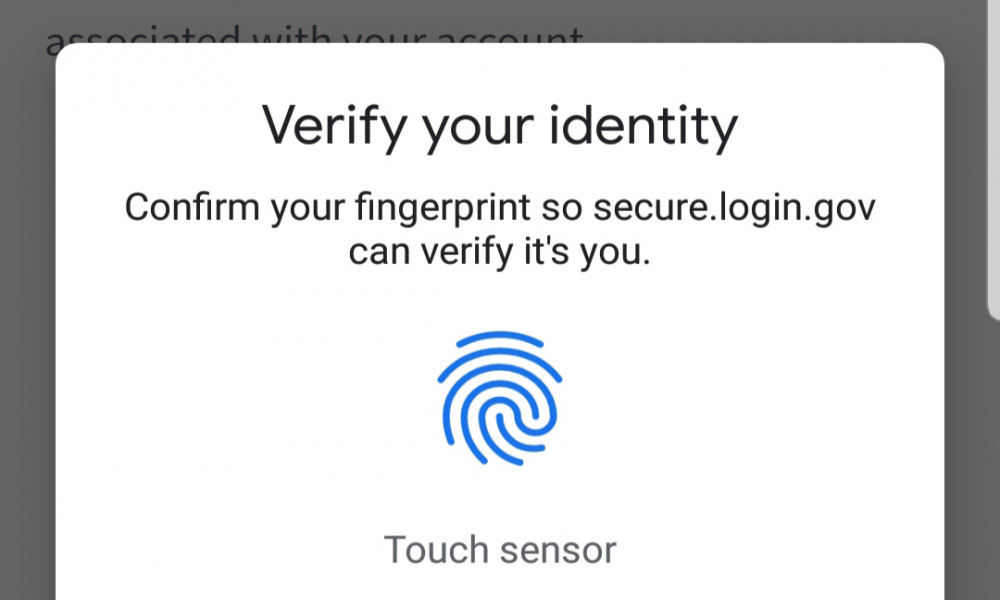