Bounded Service (Dịch vụ ràng buộc) là gì? Ví dụ xây dựng app dự báo thời tiết sử dụng Service
Ở bài viết này ta tìm hiểu về Bounded Service (Dịch vụ ràng buộc) và làm một ví dụ minh họa app dự báo thời tiết với đầu vào là vị trí địa lý, kết quả là trả về mưa hay nắng.
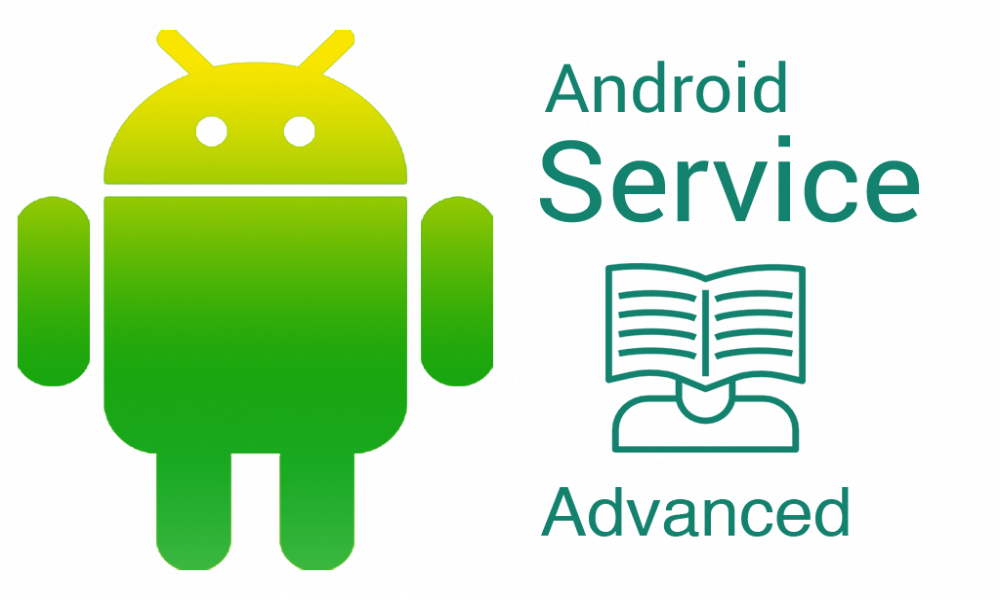
1. Bounded Service ( Dịch vụ ràng buộc) là gì?
Một Service được ràng buộc (bound) khi một thành phần ứng dụng ràng buộc nó bằng cách gọi bindService().
Một Service ràng buộc cung cấp một giao diện client-server cho phép các thành phần tương tác với Service, gửi các yêu cầu, nhận kết quả, và thậm chí làm như vậy xuyên qua nhiều tiến trình với Interprocess communication (IPC) (Truyền thông nhiều tiến trình).
Vòng đời: bạn có thể xem chi tiết ở bài viết này
2. Ví dụ app dự báo thời tiết
Ở đây tôi mô phỏng một dịch vụ cung cấp thông tin thời tiết cho ngày hiện tại, với đầu vào là vị trí địa lý (Hanoi, Chicago, ...), kết quả trả về là mưa, nắng,...
Tạo một project có tên WeatherService.
- Name: WeatherService
- Package name: org.o7planning.weatherservice
Thiết kế giao diện cho ứng dụng:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="38dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="17dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Location:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_location"
android:layout_width="0dp"
android:layout_height="47dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="23dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView_weather"
android:layout_width="0dp"
android:layout_height="45dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="59dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_location" />
<Button
android:id="@+id/button_weather"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="35dp"
android:text="Show Weather"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_weather" />
</androidx.constraintlayout.widget.ConstraintLayout>
Tạo Service:
Nhấn phải chuột vào một java package chọn:
- New > Service > Service
Nhập vào:
- Class name: WeatherService
Lớp WeatherService đã được tạo ra, đây là class mở rộng từ class android.app.Service.
Bạn có thể nhìn thấy WeatherService đã được khai báo với AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.weatherservice">
<application ...>
<service
android:name=".WeatherService"
android:enabled="true"
android:exported="true"></service>
...
</application>
</manifest>
WeatherService.java
package org.o7planning.weatherservice;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.Binder;
import android.util.Log;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
public class WeatherService extends Service {
private static String LOG_TAG = "WeatherService";
// Store the weather data.
private static final Map<String, String> weatherData = new HashMap<String,String>();
private final IBinder binder = new LocalWeatherBinder();
public class LocalWeatherBinder extends Binder {
public WeatherService getService() {
return WeatherService.this;
}
}
public WeatherService() {
}
@Override
public IBinder onBind(Intent intent) {
Log.i(LOG_TAG,"onBind");
return this.binder;
}
@Override
public void onRebind(Intent intent) {
Log.i(LOG_TAG, "onRebind");
super.onRebind(intent);
}
@Override
public boolean onUnbind(Intent intent) {
Log.i(LOG_TAG, "onUnbind");
return true;
}
@Override
public void onDestroy() {
super.onDestroy();
Log.i(LOG_TAG, "onDestroy");
}
// Returns the weather information corresponding to the location of the current date.
public String getWeatherToday(String location) {
Date now= new Date();
DateFormat df= new SimpleDateFormat("dd-MM-yyyy");
String dayString = df.format(now);
String keyLocAndDay = location + "$"+ dayString;
String weather= weatherData.get(keyLocAndDay);
//
if(weather != null) {
return weather;
}
//
String[] weathers = new String[]{"Rainy", "Hot", "Cool", "Warm" ,"Snowy"};
// Random value from 0 to 4
int i= new Random().nextInt(5);
weather =weathers[i];
weatherData.put(keyLocAndDay, weather);
//
return weather;
}
}
MainActivity.java
package org.o7planning.weatherservice;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.IBinder;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private boolean binded = false;
private WeatherService weatherService;
private TextView textViewWeather;
private EditText editTextLocation;
private Button buttonWeather;
ServiceConnection weatherServiceConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
WeatherService.LocalWeatherBinder binder = (WeatherService.LocalWeatherBinder) service;
weatherService = binder.getService();
binded = true;
}
@Override
public void onServiceDisconnected(ComponentName name) {
binded = false;
}
};
// When the Activity creating its interface.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textViewWeather = (TextView) this.findViewById(R.id.textView_weather);
this.editTextLocation = (EditText) this.findViewById(R.id.editText_location);
this.buttonWeather = (Button) this.findViewById(R.id.button_weather);
this.buttonWeather.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showWeather();
}
});
}
// When Activity starting.
@Override
protected void onStart() {
super.onStart();
// Create Intent object for WeatherService.
Intent intent = new Intent(this, WeatherService.class);
// Call bindService(..) method to bind service with UI.
this.bindService(intent, weatherServiceConnection, Context.BIND_AUTO_CREATE);
}
// Activity stop
@Override
protected void onStop() {
super.onStop();
if (binded) {
// Unbind Service
this.unbindService(weatherServiceConnection);
binded = false;
}
}
// When user click on 'Show weather' button.
public void showWeather() {
String location = this.editTextLocation.getText().toString();
String weather= this.weatherService.getWeatherToday(location);
this.textViewWeather.setText(weather);
}
}
OK, giờ bạn có thể chạy ứng dụng.
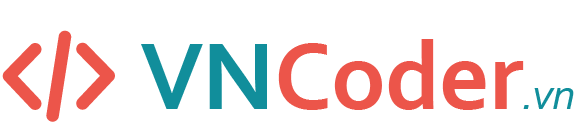
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết liên quan
Bài viết mới
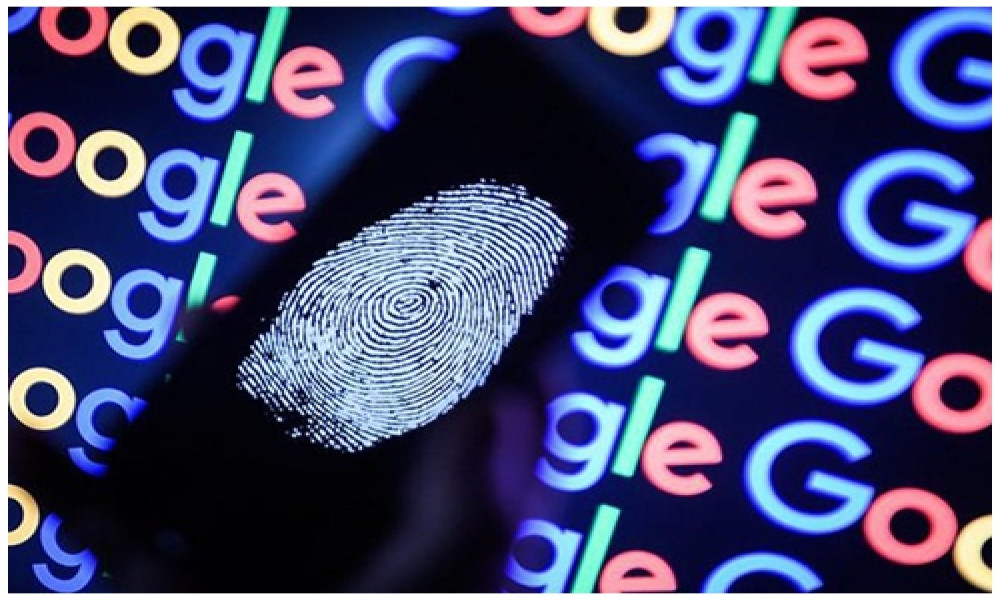
Hướng dẫn xây dựng ứng dụng sử dụng API vân tay (FINGERPRINT API) để đăng nhập, đăng ký người dùng trong Android (P2)
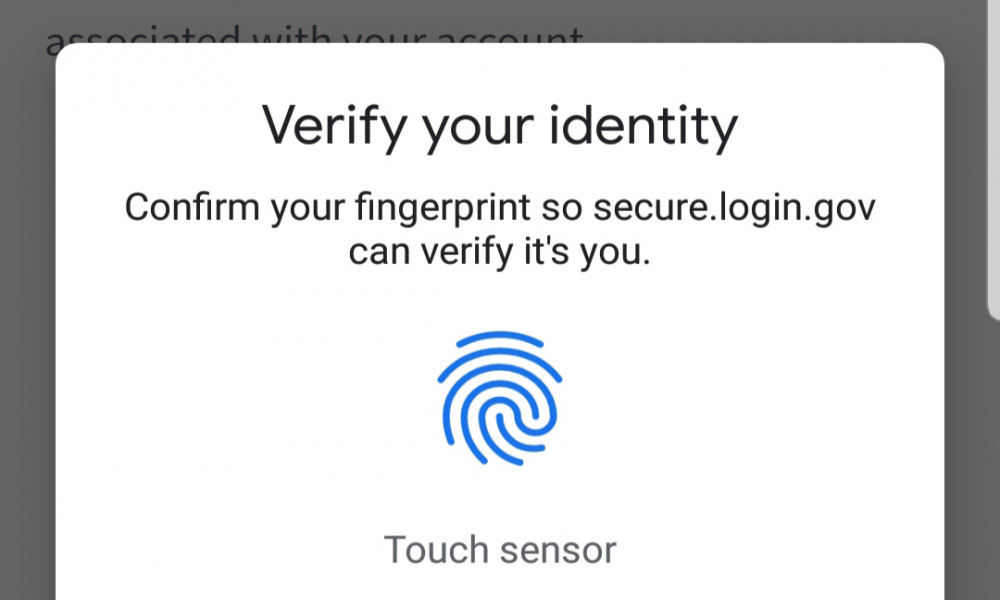