UnBuonded Service - Started Service (Service không ràng buộc) là gì? Ví dụ xây dựng app nghe nhạc (Music) chạy ngầm sử dụng Service
Trong bài viết này chúng ta sẽ đi tìm hiểu về Unbounded Service, vòng đời, cách sử dụng và ví dụ minh họa bằng một app nghe nhạc sử dụng Unbounded Service.
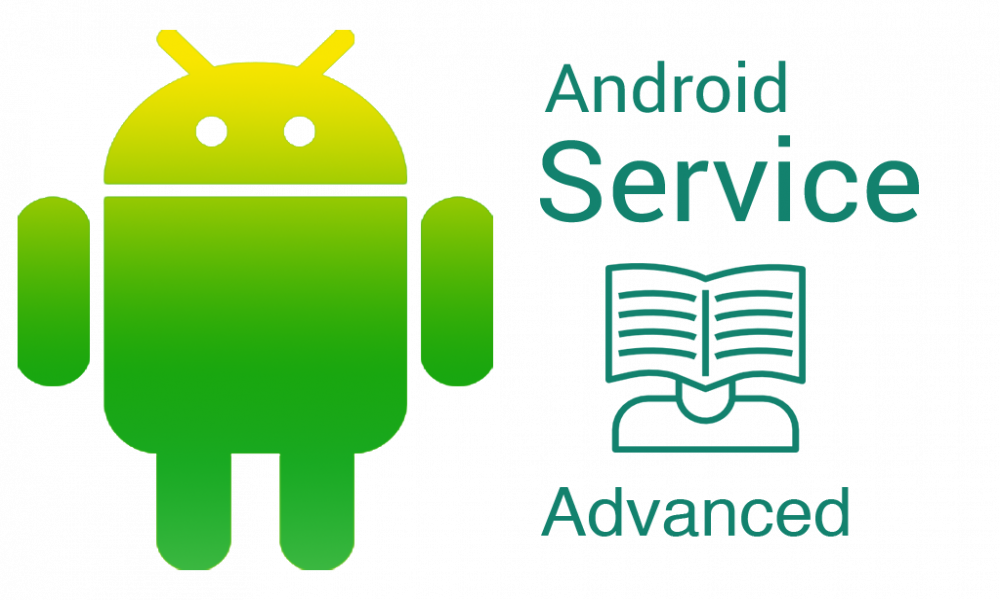
1. Unbounded Service hay còn gọi là Started Service (Service không ràng buộc) là gì?
Unbound Service (hoặc còn gọi là Started Service): Trong trường hợp này, một thành phần ứng dụng khởi động Service bằng cách gọi startService(), và Service sẽ tiếp tục chạy trong nền (background), ngay cả khi các thành phần khởi tạo nó bị phá hủy. Ví dụ, khi được bắt đầu, một Service sẽ tiếp tục chơi nhạc trong nền vô thời hạn.
2. Vòng đời Unbounded Service
Phương thức onStartCommand() trả về kiểu integer, và là một trong các giá trị sau:
- START_STICKY
- START_NOT_STICKY
- TART_REDELIVER_INTENT
START_STICKY & START_NOT_STICKY
-
Cả hai giá trị này chỉ thích hợp khi điện thoại hết bộ nhớ và hủy các Service trước khi nó kết thúc thực hiện.
-
START_STICKY nói với các hệ điều hành để tạo lại các Service sau khi đã có đủ bộ nhớ và gọi onStartCommand() một lần nữa với một Intent null.
-
START_NOT_STICKY nói với các hệ điều hành để không bận tâm tái tạo các Service một lần nữa.
Ngoài ra còn có một START_REDELIVER_INTENT giá trị thứ ba mà nói với các hệ điều hành để tạo lại các Service và truyền một Intent tương tự cho onStartCommand().
3. Ví dụ chơi nhạc (chạy ngầm) sử dụng Service
Tạo mới một "Empty Activity" project với tên PlaySongService
- Name: PlaySongService
- Package name: org.o7planning.playsongservice
Project đã được tạo ra.
Chuẩn bị file nhạc mp3:
Nhấn phải chuột vào thư mục res chọn:
- New > Folder > Raw Resources Folder
Copy và Paste một file nhạc mp3 vào thư mục 'raw' bạn vừa tạo ra.
Thiết kế giao diện của ứng dụng:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_play"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="28dp"
android:text="Play"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_stop"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="37dp"
android:text="Stop"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_play" />
</androidx.constraintlayout.widget.ConstraintLayout>
Tạo lớp Service
Nhấn phải chuột vào một java package, chọn:
- New > Service > Service
Nhập vào tên lớp:
- PlaySongService
Bạn có thể nhìn thấy PlaySongService đã được khai báo với AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.playsongservice">
<application ...>
<service
android:name=".PlaySongService"
android:enabled="true"
android:exported="true"></service>
....
</application>
</manifest>
PlaySongService.java
package org.o7planning.playsongservice;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.media.MediaPlayer;
public class PlaySongService extends Service {
private MediaPlayer mediaPlayer;
public PlaySongService() {
}
// Return the communication channel to the service.
@Override
public IBinder onBind(Intent intent){
// This service is unbounded
// So this method is never called.
return null;
}
@Override
public void onCreate(){
super.onCreate();
// Create MediaPlayer object, to play your song.
mediaPlayer = MediaPlayer.create(getApplicationContext(), R.raw.mysong);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId){
// Play song.
mediaPlayer.start();
return START_STICKY;
}
// Destroy
@Override
public void onDestroy() {
// Release the resources
mediaPlayer.release();
super.onDestroy();
}
}
MainActivity.java
package org.o7planning.playsongservice;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Intent;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private Button buttonPlay;
private Button buttonStop;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonPlay = (Button) this.findViewById(R.id.button_play);
this.buttonStop = (Button) this.findViewById(R.id.button_stop);
this.buttonPlay.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playSong();
}
});
this.buttonStop.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
stopSong();
}
});
}
// This method is called when users click on the Play button.
public void playSong() {
// Create Intent object for PlaySongService.
Intent myIntent = new Intent(MainActivity.this, PlaySongService.class);
// Call startService with Intent parameter.
this.startService(myIntent);
}
// This method is called when users click on the Stop button.
public void stopSong( ) {
// Create Intent object
Intent myIntent = new Intent(MainActivity.this, PlaySongService.class);
this.stopService(myIntent);
}
}
OK bây giờ bạn có thể chạy ứng dụng của mình và thưởng thức bài hát.
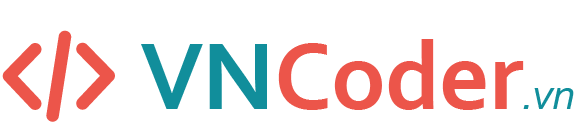
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
Bài viết liên quan
Bài viết mới
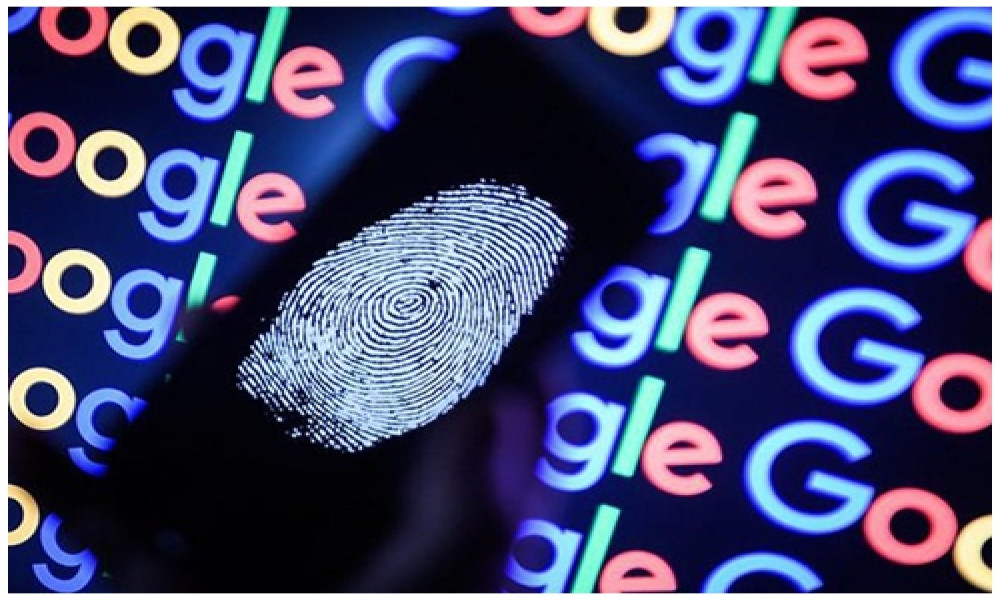
Hướng dẫn xây dựng ứng dụng sử dụng API vân tay (FINGERPRINT API) để đăng nhập, đăng ký người dùng trong Android (P2)
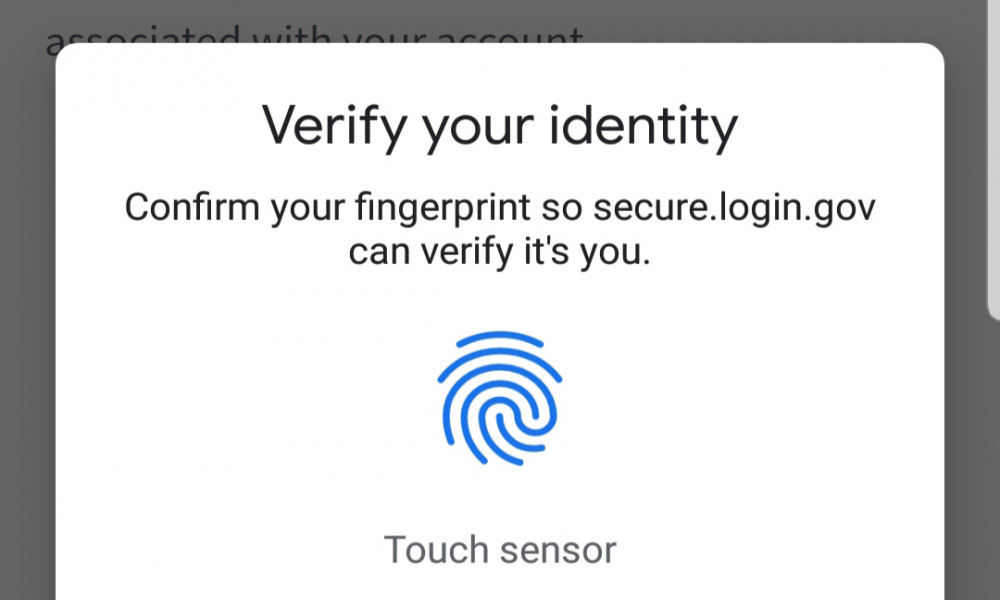