- Bài 1: Tổng quan về hệ điều hành Android
- Bài 2: Lập trình Android với Android studio
- Bài 3: Các thành phần cơ bản trong một ứng dụng Android
- Bài 4: Activity
- Bài 5: Fragment
- Bài 6: Các thành phần giao diện cơ bản trong Android
- Bài 7: Layout trong Android : Phần 1
- Bài 8: Layout trong Android : Phần 2
- Bài 9: Style và Theme trong Android
- Bài 10: Listview trong Android
- Bài 11: RecyclerView trong Android
- Bài 12: Menu trong Android
- Bài 13: Sử dụng Dialog trong Android
- Bài 14: AndroidManifest.xml Trong Android
- Bài 15: Các tài nguyên và ứng dụng cơ bản trong Android
- Bài 16: Intent trong Android
- Bài 17: Lưu trữ dữ liệu trong Android
- Bài 18: Service trong Android
- Bài 19: Content provider trong Android
- Bài 20: Broadcast Receivers trong Android
- Bài 21: SQLite trong Android
- Bài 22: Android notification
- Bài 23: Animation trong Android
- Bài 24: Android Drawables
- Bài 25: Room trong Android
- Bài 26: CursorLoader trong Android
- Bài 27: Databinding trong Android
- Bài 28: Toolbar, ActionBar trong lập trình Android
- Bài 29: AsyncTask – thread & handler trong Android
- Bài 30: Các thư viện thường dùng trong Android
- Bài 31: Tìm hiểu về MVC, MVP và MVVM
- Bài 32: AlarmManager trong Android
- Bài 33: Permission trong Android
- Bài 34: Đóng gói ứng dụng Android
Bài 23: Animation trong Android - Lập trình Android cơ bản
Đăng bởi: Admin | Lượt xem: 2948 | Chuyên mục: Android
1. Giới thiệu
Animation trong android có thể được thực hiện bằng nhiều cách. Trong bài viết này chúng tôi sẽ trình bày một cách tạo animation trong android được sử dụng rộng rãi và dễ dàng nhất gọi là Tween Animation.
Animation trong android – Tween Animation
Tween animation có một số tham số như start value , end value, size , time duration , rotation angle, … và thực hiện animation trên đối tượng. Nó có thể được áp dụng cho bất kỳ đối tượng nào. Để sử dụng Tween animation, Android cung cấp một lớp được gọi là Animation.
Để thực hiện animation trong android, chúng ta sẽ gọi một phương thức tĩnh của lớp AnimationUtils đó là loadAnimation() theo cú pháp sau
Animation animation = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.myanimation);
Lưu ý tham số thứ hai là tên của tập tin xml được sử dụng để tạo animation. Chúng ta phải tạo một thư mục mới có tên gọi anim trong thư mục res và tạo một file xml trong thư mục anim này.
Một số phương thức thường dùng của lớp Animation
STT | Phương thức và miêu tả |
---|---|
1 | start() Bắt đầu animation. |
2 | setDuration(long duration) Thiết lập thời gian của một animation. |
3 | end() Kết thúc animation. |
4 | cancel() Huỷ animation. |
Để áp dụng animation cho một đối tượng, chúng ta sẽ gọi phương thức startAnimation() của đối tượng muốn áp dụng theo cú pháp sau
ImageView iv = (ImageView)findViewById(R.id.iv);
iv.startAnimation(animation);
Trong đó iv là đối tượng mà bạn muốn thiết lập animation
Một số animation thông dụng
Mỗi một animation sẽ tương ứng với một file xml và có thể liệt kê một số animation thông dụng sau:
- Fade In
- Fade Out
- Blink
- Zoom In
- Zoom Out
- Rotate
- Move
- Slide Up
- Slide Down
- Sequential
- Together
Các thuộc tính quan trọng của tập tin XML
Thuộc tính | Miêu tả |
---|---|
android:duration | Thời gian hoàn thành |
android:startOffset | Thời gian chờ trước khi một animaiton bắt đầu và thường được sử dụng khi có nhiều animation |
android:repeatMode | Thiết lập lặp lại animation |
android:repeatCount | Xác định số lần lặp lại animation. Nếu bạn thiết lập giá trị này là infinite thì animation sẽ lặp lại lần vô hạn |
android:interpolator | Tỷ lệ thay đổi animation |
android:fillAfter | Xác định liệu có áp dụng việc chuyển đổi đối tượng về trạng thái ban đầu sau khi một animation đã hoàn thành hay không. |
Animation trong android – Fade In (Hiệu ứng mờ)
Sử dụng thẻ <alpha> để định nghĩa giá trị alpha và giá trị alpha sẽ được tăng từ 0 đến 1
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="1000"
android:fromAlpha="0.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="1.0" />
</set>
Animation trong android – Fade Out (Hiệu ứng mờ)
Ngược lại với Fade In và giá trị alpha sẽ được thay đổi từ 1 đến 0
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="1000"
android:fromAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="0.0" />
</set>
Animation trong android – Blink (Hiệu ứng nhấp nháy)
Là một fade in hoặc fade out và được lặp đi lặp lại. Để thực hiện điều này chúng ta phải thiết lập android:repeatMode=”reverse” và android:repeatCount=”infinite”
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<alpha android:fromAlpha="0.0"
android:toAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:duration="600"
android:repeatMode="reverse"
android:repeatCount="infinite"/>
</set>
Animation trong android – Zoom In (Hiệu ứng phóng to)
Sử dụng thẻ <scale>. Sử dụng pivotX=”50%” và pivotY=”50%” để thực hiện zoom từ trung tâm của đối tượng. Ngoài ra chúng ta cần sử dụng fromXScale và fromYScale để qui định tỷ lệ phóng to đối tượng. Chỉ định giá trị cho các thuộc tính này phải nhỏ hơn toXScale và toYScale
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:fromXScale="1"
android:fromYScale="1"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="3"
android:toYScale="3" >
</scale>
</set>
Animation trong android – Zoom Out (Hiệu ứng thu nhỏ)
Cũng tương tự Zoom Out nhưng giá trị của toXScale và toYScale được chỉ định thấp hơn fromXScale và fromYScale
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="0.5"
android:toYScale="0.5" >
</scale>
</set>
Animation trong android – Rotate (Hiệu ứng quay)
Hiệu ứng Rotate sử dụng thẻ <rotate> và yêu cầu sử dụng android:fromDegrees và android:toDegrees để xác định góc quay. Nếu chỉ định giá trị dương cho thuộc tính toDegrees thì sẽ quay theo chiều kim đồng hồ và ngược lại.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<rotate android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:duration="600"
android:repeatMode="restart"
android:repeatCount="infinite"
android:interpolator="@android:anim/cycle_interpolator"/>
</set>
Animation trong android – Move (Hiệu ứng di chuyển)
Để thay đổi vị trí của đối tượng chúng ta sử dụng thẻ <translate>. Sử dụng các thuộc tính android:fromXDelta, android:fromYDelta cho hướng X và android:toXDelta, android:toYDelta cho hướng Y.
<?xml version="1.0" encoding="utf-8"?>
<set
xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator"
android:fillAfter="true">
<translate
android:fromXDelta="0%p"
android:toXDelta="75%p"
android:duration="800" />
</set>
Animation trong android – Slide Up (Hiệu ứng trượt lên)
Sử dụng thẻ <scale> kết hợp với 2 thuộc tính android:fromYScale=”1.0″ và android:toYScale=”0.0″
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:interpolator="@android:anim/linear_interpolator"
android:toXScale="1.0"
android:toYScale="0.0" />
</set>
Animation trong android – Slide Down (Hiệu ứng trượt xuống)
Ngược lại với Slide Up, thiết lập giá trị cho 2 thuộc tính android:fromYScale=”0.0″ và android:toYScale=”1.0″
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:interpolator="@android:anim/linear_interpolator"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
Animation trong android – Sequential Animation (Hiệu ứng thực hiện tuần tự)
Nếu muốn thực hiện nhiều animation một cách tuần tự, chúng ta phải sử dụng android:startOffset để thiết lập thời gian trễ. Cách đơn giản để tính toán giá trị cho android:startOffset là cộng giá trị android:duration của hiệu ứng hiện tại với giá trị android:startOffset của hiệu ứng trước
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true"
android:interpolator="@android:anim/linear_interpolator" >
<!-- Use startOffset to give delay between animations -->
<!-- Move -->
<translate
android:duration="800"
android:fillAfter="true"
android:fromXDelta="0%p"
android:startOffset="300"
android:toXDelta="75%p" />
<translate
android:duration="800"
android:fillAfter="true"
android:fromYDelta="0%p"
android:startOffset="1100"
android:toYDelta="70%p" />
<translate
android:duration="800"
android:fillAfter="true"
android:fromXDelta="0%p"
android:startOffset="1900"
android:toXDelta="-75%p" />
<translate
android:duration="800"
android:fillAfter="true"
android:fromYDelta="0%p"
android:startOffset="2700"
android:toYDelta="-70%p" />
<!-- Rotate 360 degrees -->
<rotate
android:duration="1000"
android:fromDegrees="0"
android:interpolator="@android:anim/cycle_interpolator"
android:pivotX="50%"
android:pivotY="50%"
android:startOffset="3700"
android:repeatCount="infinite"
android:repeatMode="restart"
android:toDegrees="360" />
</set>
Animation trong android – Together Animation (Hiệu ứng thực hiện đồng thời)
Viết tất cả các hiệu ứng mà không chỉ định android:startOffset
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true"
android:interpolator="@android:anim/linear_interpolator" >
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="4000"
android:fromXScale="1"
android:fromYScale="1"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="4"
android:toYScale="4" >
</scale>
<!-- Rotate 180 degrees -->
<rotate
android:duration="500"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:repeatCount="infinite"
android:repeatMode="restart"
android:toDegrees="360" />
</set>
Animation trong android – Các bước thực hiện
Bước 1: Tạo tập tin xml định nghĩa animation (Tập tin này được đặt trong thư mục anim dưới thư mục res (res ⇒ anim ⇒ xml))
1/ Tạo thư mục anim bằng cách chuột phải thư mục res -> chọn New -> chọn Directory
2/ Tạo tập tin xml bằng cách chuột phải thư mục anim -> chọn New -> chọn Animation resource file
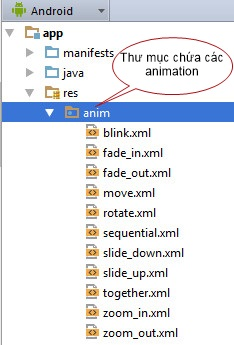
Bước 2: Nạp animation
Tại activity tạo một đối tượng của lớp Animation và nạp tập tin xml sử dụng phương thức loadAnimation() của lớp AnimationUtils
Bước 3: Thiết lập sự kiện (Tuỳ chọn)
Nếu bạn muốn xử lý các sự kiện như start, end và repeat, bạn phải cài đặt giao diện AnimationListener cho activity của bạn. Lưu ý bước này là tuỳ chọn, bạn có thể bỏ qua nếu không cần thiết.
Bước 4: Bắt đầu animation
Chúng ta có thể bắt đầu một animation bất cứ khi nào mình muốn bằng cách gọi phương thức startAnimation() trên bất kỳ thành phần UI nào mà chúng ta muốn thiết lập animation
Animation trong android – Ví dụ thiết lập hiệu ứng Fade In cho thành phần UI là TextView
Bước 1: Tạo tập tin fade_in.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="1000"
android:fromAlpha="0.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="1.0" />
</set>
Bước 2, 3 & 4: Tạo activity SampleAnimationActivity, nạp tập tin xml, đăng ký sự kiện và bắt đầu animation
Thiết kế giao diện
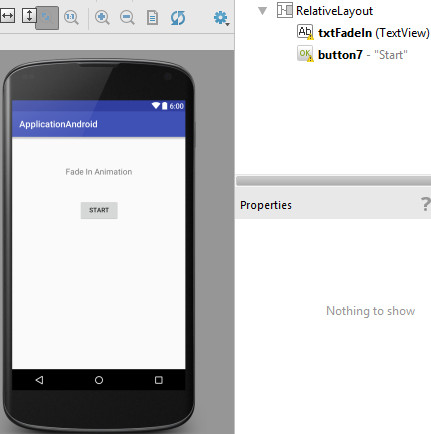
Viết xử lý
public class SampleAnimationActivity extends AppCompatActivity implements Animation.AnimationListener{
TextView txtMessage;
// Animation
Animation animFadein;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sample_animation);
txtMessage = (TextView) findViewById(R.id.txtFadeIn);
// load the animation
animFadein = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.fade_in);
// set animation listener
animFadein.setAnimationListener(this);
}
public void startAnimation(View view){
// start the animation
txtMessage.startAnimation(animFadein);
}
@Override
public void onAnimationStart(Animation animation) {
// Animation started
}
@Override
public void onAnimationEnd(Animation animation) {
Toast.makeText(getApplicationContext(), "Animation Stopped", Toast.LENGTH_SHORT).show();
}
@Override
public void onAnimationRepeat(Animation animation) {
// Animation is repeating
}
}
Animation trong android – Bài tập thực hành
Thiết kế giao diện của ứng dụng và thực hiện animationcho thành phần ImageView
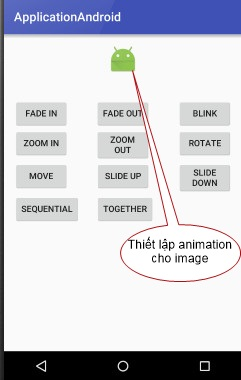
Hi vọng bài viết giúp ích ít nhiều trong công việc của các bạn.
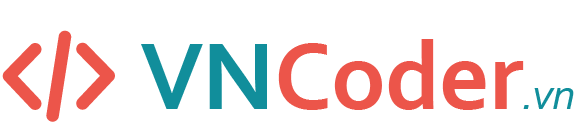
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
- Bài 1: Tổng quan về hệ điều hành Android
- Bài 2: Lập trình Android với Android studio
- Bài 3: Các thành phần cơ bản trong một ứng dụng Android
- Bài 4: Activity
- Bài 5: Fragment
- Bài 6: Các thành phần giao diện cơ bản trong Android
- Bài 7: Layout trong Android : Phần 1
- Bài 8: Layout trong Android : Phần 2
- Bài 9: Style và Theme trong Android
- Bài 10: Listview trong Android
- Bài 11: RecyclerView trong Android
- Bài 12: Menu trong Android
- Bài 13: Sử dụng Dialog trong Android
- Bài 14: AndroidManifest.xml Trong Android
- Bài 15: Các tài nguyên và ứng dụng cơ bản trong Android
- Bài 16: Intent trong Android
- Bài 17: Lưu trữ dữ liệu trong Android
- Bài 18: Service trong Android
- Bài 19: Content provider trong Android
- Bài 20: Broadcast Receivers trong Android
- Bài 21: SQLite trong Android
- Bài 22: Android notification
- Bài 23: Animation trong Android
- Bài 24: Android Drawables
- Bài 25: Room trong Android
- Bài 26: CursorLoader trong Android
- Bài 27: Databinding trong Android
- Bài 28: Toolbar, ActionBar trong lập trình Android
- Bài 29: AsyncTask – thread & handler trong Android
- Bài 30: Các thư viện thường dùng trong Android
- Bài 31: Tìm hiểu về MVC, MVP và MVVM
- Bài 32: AlarmManager trong Android
- Bài 33: Permission trong Android
- Bài 34: Đóng gói ứng dụng Android