- Bài 1: ReactJS - Lời mở đầu
- Bài 2: ReactJS - Thiết lập môi trường
- Bài 3: ReactJS - JSX
- Bài 4: ReactJS - Components & State
- Bài 5: Props - Tổng quan
- Bài 6: Props - Validation
- Bài 7: ReactJJS - Component API
- Bài 8: ReactJS - Vòng đời của Component
- Bài 9: ReactJS - Forms
- Bài 10: ReactJS - Xử lý sự kiện
- Bài 11: ReactJS - Refs
- Bài 12: ReactJS - List và Keys
- Bài 13: ReactJS - Router
- Bài 14: ReactJS - Khái niệm Flux
- Bài 15: ReactJS - Cách sử dụng Flux
- Bài 16: ReactJS - Animation
- Bài 17: ReactJS - Higher Order Components
- Bài 18: ReactJS - Bản rút gọn(Quick guide - P1)
- Bài 19: ReactJS - Bản rút gọn(Quick guide - P2)
- Bài 20: ReactJS - Bản rút gọn(Quick guide - P3)
- Bài 21: ReactJS - Tạo ứng dụng reatjs từ bootstrap 4
Bài 15: ReactJS - Cách sử dụng Flux - Học ReactJS Full Đầy Đủ Nhất
Đăng bởi: Admin | Lượt xem: 1612 | Chuyên mục: Javascript
1. Cài đặt Redux
Bản chất làm việc với React là việc truyền dữ liệu giữa các component và thay đổi state để rerender lại giao diện component. Nếu dự án chúng ta lớn, việc truyền props từ Component cha sang nhiều component con khác sẽ rất khó để theo dõi code và bảo trì code.
Redux là thư viện cung cấp cho ta một store trung tâm, lưu trữ tất cả các state, từ component muốn thay đổi state chỉ cần truy cập tới store để thay đổi.
Chúng ta sẽ cài đặt Redux theo dòng lệnh sau :
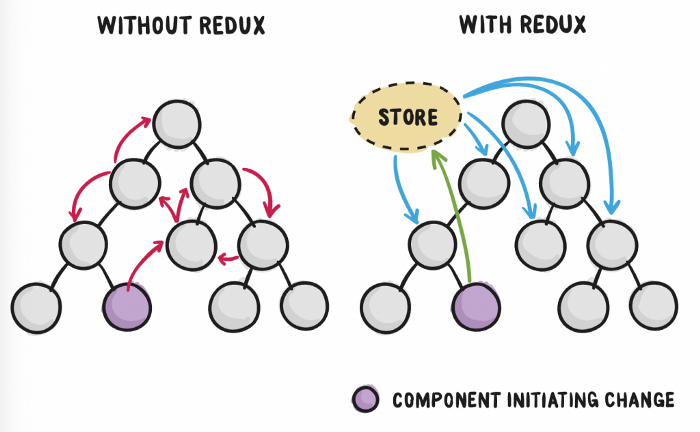
install --save react-redux
2. Tạo files và folders :
Trong bước nàym ta sẽ tạo foldes và files với các actions, reducers, và components. Sau khi hoàn thành, chúng ta tiến hành cài đặt cấu trúc thư mục như sau :
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir actions
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir components
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir reducers
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > actions/actions.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > reducers/reducers.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/AddTodo.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/Todo.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/TodoList.js
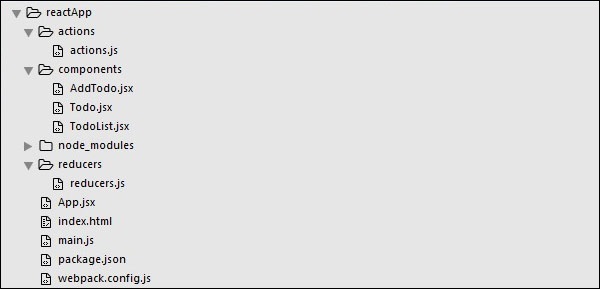
3. Actions :
Actions là các đối tượng JavaScript sử dụng thuộc tính kiểu để thông báo về dữ liệu sẽ được gửi đến nơi lưu trữ. Mình sử dụng ADD_TODO để thêm mục mới vào danh sách Hàm addTodo trả về hành động và đặt một id cho mọi mục đã tạo.
actions/actions.js
export const ADD_TODO = 'ADD_TODO'
let nextTodoId = 0;
export function addTodo(text) {
return {
type: ADD_TODO,
id: nextTodoId++,
text
};
}
4. Reducers
Reducer chắc chắn là khái niệm quan trọng nhất trong Redux. Nó sản xuất state của ứng dụng.Nhưng làm thế nào để một reducer biết khi nào sẽ tạo ra state tiếp theo. Nguyên tắc thứ 2 của Redux cho biết cách duy nhất để thay đổi state là gửi các tín hiệu đến store. Tín hiệu này là một hành động (action). “Dispatching an action” là quá trình để gửi tín hiệu.
Bây giờ làm thế nào để thay đổi state bất biến. State là kết quả của một bản sao của state hiện tại và dữ liệu mới.
Điều đáng quan tâm ở đây là các hành động (action) redux là một đối tượng Javascript. Dưới đây là một hành động.
Mặc dù các hành động chỉ kích hoạt các thay đổi trong ứng dụng, nhưng bộ giảm chỉ định các thay đổi đó. đang sử dụng câu lệnh switch để tìm kiếm hành động ADD_TODO. Bộ giảm là một hàm nhận hai tham số (trạng thái và hành động) để tính toán và trả về trạng thái cập nhật.
Chức năng đầu tiên sẽ được sử dụng để tạo một mục mới, trong khi chức năng thứ hai sẽ đẩy mục đó vào danh sách. Cuối cùng, chúng tôi đang sử dụng chức năng trợ giúp connectReducers nơi chúng tôi có thể thêm bất kỳ bộ giảm mới nào mà chúng tôi có thể sử dụng trong tương lai.
reducers/reducers.js
import { combineReducers } from 'redux'
import { ADD_TODO } from '../actions/actions'
function todo(state, action) {
switch (action.type) {
case ADD_TODO:
return {
id: action.id,
text: action.text,
}
default:
return state
}
}
function todos(state = [], action) {
switch (action.type) {
case ADD_TODO:
return [
...state,
todo(undefined, action)
]
default:
return state
}
}
const todoApp = combineReducers({
todos
})
export default todoApp
5. Store
Store là nơi lưu giữ trạng thái của ứng dụng. Rất dễ dàng để tạo một store sau khi bạn có reducers.Mình đang chuyển thuộc tính store cho phần tử cung cấp, phần tử này bao bọc thành phần router.
import React from 'react'
import { render } from 'react-dom'
import { createStore } from 'redux'
import { Provider } from 'react-redux'
import App from './App.jsx'
import todoApp from './reducers/reducers'
let store = createStore(todoApp)
let rootElement = document.getElementById('app')
render(
<Provider store = {store}>
<App />
</Provider>,
rootElement
)
6. Root Component
App component là một component gốc của ứng dụng. Chỉ có component gốc nên biết về redux. Điều qua trọng để lưu ý là hàm connect chỉ sử dụng khi kết nối component gốc App với store
Hàm này sẽ sử dụng hàm select như là thuật toán. Hàm select lấy trạng thái từ nơi lưu trữ và trả về props(visibleTodos) mà ta có thể sử dụng trong các components
App.jsx
import React, { Component } from 'react'
import { connect } from 'react-redux'
import { addTodo } from './actions/actions'
import AddTodo from './components/AddTodo.js'
import TodoList from './components/TodoList.js'
class App extends Component {
render() {
const { dispatch, visibleTodos } = this.props
return (
<div>
<AddTodo onAddClick = {text =>dispatch(addTodo(text))} />
<TodoList todos = {visibleTodos}/>
</div>
)
}
}
function select(state) {
return {
visibleTodos: state.todos
}
}
export default connect(select)(App);
7. Các component khác
Các component này không nên sử dụng redux
AddTodo.js
import React, { Component, PropTypes } from 'react'
export default class AddTodo extends Component {
render() {
return (
<div>
<input type = 'text' ref = 'input' />
<button onClick = {(e) => this.handleClick(e)}>
Add
</button>
</div>
)
}
handleClick(e) {
const node = this.refs.input
const text = node.value.trim()
this.props.onAddClick(text)
node.value = ''
}
}
Todo.js
import React, { Component, PropTypes } from 'react'
export default class Todo extends Component {
render() {
return (
<li>
{this.props.text}
</li>
)
}
}
TodoList.js
import React, { Component, PropTypes } from 'react'
import Todo from './Todo.js'
export default class TodoList extends Component {
render() {
return (
<ul>
{this.props.todos.map(todo =>
<Todo
key = {todo.id}
{...todo}
/>
)}
</ul>
)
}
}
Mở terminal và chạy App, sau khi chạy xong thì ta được kết quả như sau :
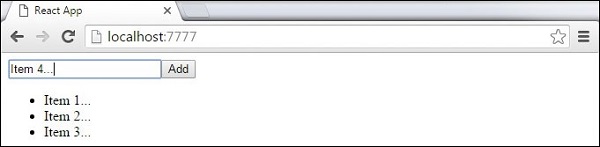
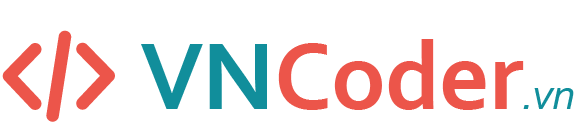
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
- Bài 1: ReactJS - Lời mở đầu
- Bài 2: ReactJS - Thiết lập môi trường
- Bài 3: ReactJS - JSX
- Bài 4: ReactJS - Components & State
- Bài 5: Props - Tổng quan
- Bài 6: Props - Validation
- Bài 7: ReactJJS - Component API
- Bài 8: ReactJS - Vòng đời của Component
- Bài 9: ReactJS - Forms
- Bài 10: ReactJS - Xử lý sự kiện
- Bài 11: ReactJS - Refs
- Bài 12: ReactJS - List và Keys
- Bài 13: ReactJS - Router
- Bài 14: ReactJS - Khái niệm Flux
- Bài 15: ReactJS - Cách sử dụng Flux
- Bài 16: ReactJS - Animation
- Bài 17: ReactJS - Higher Order Components
- Bài 18: ReactJS - Bản rút gọn(Quick guide - P1)
- Bài 19: ReactJS - Bản rút gọn(Quick guide - P2)
- Bài 20: ReactJS - Bản rút gọn(Quick guide - P3)
- Bài 21: ReactJS - Tạo ứng dụng reatjs từ bootstrap 4