- Bài 1: ReactJS - Lời mở đầu
- Bài 2: ReactJS - Thiết lập môi trường
- Bài 3: ReactJS - JSX
- Bài 4: ReactJS - Components & State
- Bài 5: Props - Tổng quan
- Bài 6: Props - Validation
- Bài 7: ReactJJS - Component API
- Bài 8: ReactJS - Vòng đời của Component
- Bài 9: ReactJS - Forms
- Bài 10: ReactJS - Xử lý sự kiện
- Bài 11: ReactJS - Refs
- Bài 12: ReactJS - List và Keys
- Bài 13: ReactJS - Router
- Bài 14: ReactJS - Khái niệm Flux
- Bài 15: ReactJS - Cách sử dụng Flux
- Bài 16: ReactJS - Animation
- Bài 17: ReactJS - Higher Order Components
- Bài 18: ReactJS - Bản rút gọn(Quick guide - P1)
- Bài 19: ReactJS - Bản rút gọn(Quick guide - P2)
- Bài 20: ReactJS - Bản rút gọn(Quick guide - P3)
- Bài 21: ReactJS - Tạo ứng dụng reatjs từ bootstrap 4
Bài 21: ReactJS - Tạo ứng dụng reatjs từ bootstrap 4 - Học ReactJS Full Đầy Đủ Nhất
Đăng bởi: Admin | Lượt xem: 8195 | Chuyên mục: Javascript
Trong bài này, mình sẽ hướng dẫn cho bạn cách chuyển một bootstrap 4 sang reactjs một cách đơn giản, các bước thực hiện như sau :
Bước 1 : Tạo app và các tải về bootstrap 4 :
Chạy câu lệnh sau :
npx create-react-app myapp
Download bất kì bootstrap nào bạn thích, ở đây mình sử dụng bootstrap này
Ngoài ra bạn còn sử dụng web htmltojsx để convert html sang jsx được React khuyến nghị nên sử dụng
Sau khi download bootstrap, bạn move nó vào project của mình như dưới
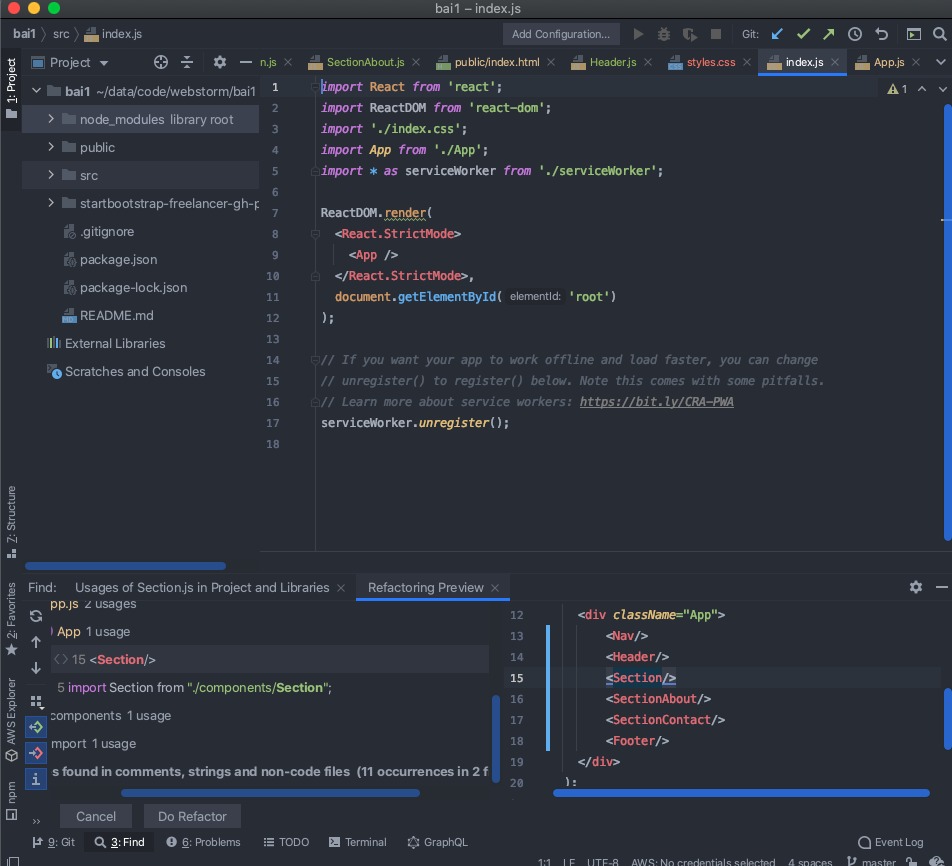
Bước 2 : Cài đặt liên kết và cấu trúc project
Sau khi di chuyển bootstrap vào project, ta tiếp tục move 3 thư mục css, assets và js vào thư mục public như sau
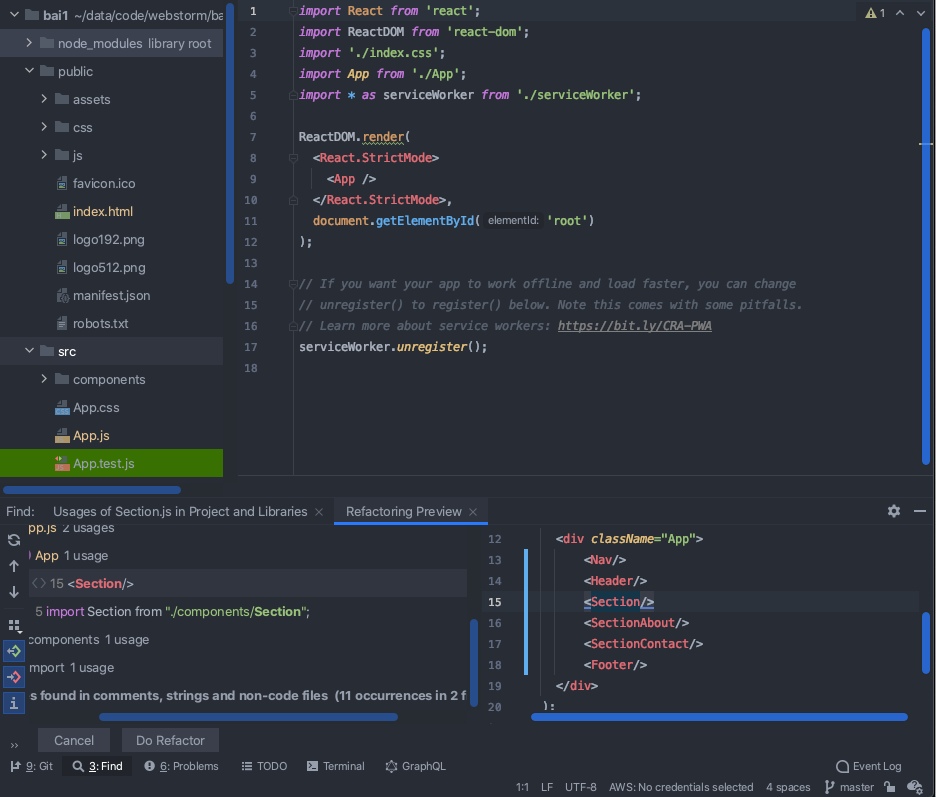
Tiếp theo, tìm đển file index.html trong bootstrap, copy các câu script và link , sau đó đến file index.html trong public , dán các dòng tương ứng, chỉnh sửa ../public thành %PUBLIC_URL%
public/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta
name="description"
content="Web site created using create-react-app"
/>
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<!--
manifest.json provides metadata used when your web app is installed on a
user's mobile device or desktop. See https://developers.google.com/web/fundamentals/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<!-- Favicon-->
<link rel="icon" type="image/x-icon" href="%PUBLIC_URL%/assets/img/favicon.ico" />
<!-- Font Awesome icons (free version)-->
<script src="https://use.fontawesome.com/releases/v5.13.0/js/all.js" crossorigin="anonymous"></script>
<!-- Google fonts-->
<link href="https://fonts.googleapis.com/css?family=Montserrat:400,700" rel="stylesheet" type="text/css" />
<link href="https://fonts.googleapis.com/css?family=Lato:400,700,400italic,700italic" rel="stylesheet" type="text/css" />
<!-- Core theme CSS (includes Bootstrap)-->
<link href="%PUBLIC_URL%/css/styles.css" rel="stylesheet" />
-->
<title>React App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.bundle.min.js"></script>
<!-- Third party plugin JS-->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-easing/1.4.1/jquery.easing.min.js"></script>
<!-- Contact form JS-->
<script src="%PUBLIC_URL%/assets/mail/jqBootstrapValidation.js"></script>
<script src="%PUBLIC_URL%/assets/mail/contact_me.js"></script>
<!-- Core theme JS-->
<script src="%PUBLIC_URL%/js/scripts.js"></script>
</html>
Bước 3 : Chuyển html sang jsx
Đây là bước quan trọng và cốt yếu của bài hôm nay, ở đây mình sẽ tạo ra các component, và sau đó chia html thành 6 components phù hợp với bố cục bootstrap
- Nav.js
- Header.js
- SectionPorfolio.js
- SectionAbout.js
- SectionContact.js
- Footer.js
Bạn tiến hành copy từng đoạn code trong html tương ứng từng phần vào dán vào page htmltojsx sao đó copy đoạn code trong jsx dáng vào các file js ở trên. Code chi tiết từng component như sau :
Nav.js
import React, {Component} from 'react';
class Nav extends Component {
render() {
return (
<nav className="navbar navbar-expand-lg bg-secondary text-uppercase fixed-top" id="mainNav">
<div className="container">
<a className="navbar-brand js-scroll-trigger" href="#page-top">Start Bootstrap</a>
<button
className="navbar-toggler navbar-toggler-right text-uppercase font-weight-bold bg-primary text-white rounded"
type="button" data-toggle="collapse" data-target="#navbarResponsive"
aria-controls="navbarResponsive" aria-expanded="false" aria-label="Toggle navigation">
Menu
<i className="fas fa-bars"></i>
</button>
<div className="collapse navbar-collapse" id="navbarResponsive">
<ul className="navbar-nav ml-auto">
<li className="nav-item mx-0 mx-lg-1"><a
className="nav-link py-3 px-0 px-lg-3 rounded js-scroll-trigger"
href="#portfolio">Portfolio</a></li>
<li className="nav-item mx-0 mx-lg-1"><a
className="nav-link py-3 px-0 px-lg-3 rounded js-scroll-trigger" href="#about">About</a>
</li>
<li className="nav-item mx-0 mx-lg-1"><a
className="nav-link py-3 px-0 px-lg-3 rounded js-scroll-trigger"
href="#contact">Contact</a></li>
</ul>
</div>
</div>
</nav>
);
}
}
export default Nav;
Header.js
import React, {Component} from 'react';
class Header extends Component {
render() {
return (
<header className="masthead bg-primary text-white text-center">
<div className="container d-flex align-items-center flex-column">
<img className="masthead-avatar mb-5" src="assets/img/avataaars.svg" alt="" />
{/* Masthead Heading*/}
<h1 className="masthead-heading text-uppercase mb-0">Trần Quân Đạt</h1>
{/* Icon Divider*/}
<div className="divider-custom divider-light">
<div className="divider-custom-line" />
<div className="divider-custom-icon"><i className="fas fa-star" /></div>
<div className="divider-custom-line" />
</div>
{/* Masthead Subheading*/}
<p className="masthead-subheading font-weight-light mb-0">Ăn - Chơi - Ngủ</p>
</div>
</header>
);
}
}
export default Header;
SectionPorfilio.js
import React, {Component} from 'react';
class Section extends Component {
render() {
return (
<section className="page-section portfolio" id="portfolio">
<div className="container">
{/* Portfolio Section Heading*/}
<h2 className="page-section-heading text-center text-uppercase text-secondary mb-0">Trình độ</h2>
{/* Icon Divider*/}
<div className="divider-custom">
<div className="divider-custom-line" />
<div className="divider-custom-icon"><i className="fas fa-star" /></div>
<div className="divider-custom-line" />
</div>
{/* Portfolio Grid Items*/}
<div className="row">
{/* Portfolio Item 1*/}
<div className="col-md-6 col-lg-4 mb-5">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal1">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/cabin.png" alt="" />
</div>
</div>
{/* Portfolio Item 2*/}
<div className="col-md-6 col-lg-4 mb-5">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal2">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/cake.png" alt="" />
</div>
</div>
{/* Portfolio Item 3*/}
<div className="col-md-6 col-lg-4 mb-5">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal3">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/circus.png" alt="" />
</div>
</div>
{/* Portfolio Item 4*/}
<div className="col-md-6 col-lg-4 mb-5 mb-lg-0">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal4">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/game.png" alt="" />
</div>
</div>
{/* Portfolio Item 5*/}
<div className="col-md-6 col-lg-4 mb-5 mb-md-0">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal5">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/safe.png" alt="" />
</div>
</div>
{/* Portfolio Item 6*/}
<div className="col-md-6 col-lg-4">
<div className="portfolio-item mx-auto" data-toggle="modal" data-target="#portfolioModal6">
<div className="portfolio-item-caption d-flex align-items-center justify-content-center h-100 w-100">
<div className="portfolio-item-caption-content text-center text-white"><i className="fas fa-plus fa-3x" /></div>
</div>
<img className="img-fluid" src="assets/img/portfolio/submarine.png" alt="" />
</div>
</div>
</div>
</div>
</section>
);
}
}
export default Section;
SectionAbout.js
import React, {Component} from 'react';
class SectionAbout extends Component {
render() {
return (
<section className="page-section bg-primary text-white mb-0" id="about">
<div className="container">
{/* About Section Heading*/}
<h2 className="page-section-heading text-center text-uppercase text-white">Về chúng tôi</h2>
{/* Icon Divider*/}
<div className="divider-custom divider-light">
<div className="divider-custom-line" />
<div className="divider-custom-icon"><i className="fas fa-star" /></div>
<div className="divider-custom-line" />
</div>
{/* About Section Content*/}
<div className="row">
<div className="col-lg-4 ml-auto"><p className="lead">Trần Quân Đạt </p></div>
<div className="col-lg-4 mr-auto"><p className="lead">Nguyễn Văn Hiếu</p></div>
</div>
{/* About Section Button*/}
<div className="text-center mt-4">
<a className="btn btn-xl btn-outline-light" href="https://google.com/">
<i className="fas fa-download mr-2" />
Xem chi tiết ở đây
</a>
</div>
</div>
</section>
);
}
}
export default SectionAbout;
SectionContact.js
import React, {Component} from 'react';class SectionContact extends Component { render() { return ( <section className="page-section" id="contact"> <div className="container"> {/* Contact Section Heading*/} <h2 className="page-section-heading text-center text-uppercase text-secondary mb-0">Contact Me</h2> {/* Icon Divider*/} <div className="divider-custom"> <div className="divider-custom-line" /> <div className="divider-custom-icon"><i className="fas fa-star" /></div> <div className="divider-custom-line" /> </div> {/* Contact Section Form*/} <div className="row"> <div className="col-lg-8 mx-auto"> {/* To configure the contact form email address, go to mail/contact_me.php and update the email address in the PHP file on line 19.*/} <form id="contactForm" name="sentMessage" noValidate="novalidate"> <div className="control-group"> <div className="form-group floating-label-form-group controls mb-0 pb-2"> <label>Name</label> <input className="form-control" id="name" type="text" placeholder="Name" required="required" data-validation-required-message="Please enter your name." /> <p className="help-block text-danger" /> </div> </div> <div className="control-group"> <div className="form-group floating-label-form-group controls mb-0 pb-2"> <label>Email Address</label> <input className="form-control" id="email" type="email" placeholder="Email Address" required="required" data-validation-required-message="Please enter your email address." /> <p className="help-block text-danger" /> </div> </div> <div className="control-group"> <div className="form-group floating-label-form-group controls mb-0 pb-2"> <label>Phone Number</label> <input className="form-control" id="phone" type="tel" placeholder="Phone Number" required="required" data-validation-required-message="Please enter your phone number." /> <p className="help-block text-danger" /> </div> </div> <div className="control-group"> <div className="form-group floating-label-form-group controls mb-0 pb-2"> <label>Message</label> <textarea className="form-control" id="message" rows={5} placeholder="Message" required="required" data-validation-required-message="Please enter a message." defaultValue={""} /> <p className="help-block text-danger" /> </div> </div> <br /> <div id="success" /> <div className="form-group"><button className="btn btn-primary btn-xl" id="sendMessageButton" type="submit">Send</button></div> </form> </div> </div> </div> </section> ); }}export default SectionContact;
Footer.js
import React, {Component} from 'react';
class SectionContact extends Component {
render() {
return (
<section className="page-section" id="contact">
<div className="container">
{/* Contact Section Heading*/}
<h2 className="page-section-heading text-center text-uppercase text-secondary mb-0">Contact Me</h2>
{/* Icon Divider*/}
<div className="divider-custom">
<div className="divider-custom-line" />
<div className="divider-custom-icon"><i className="fas fa-star" /></div>
<div className="divider-custom-line" />
</div>
{/* Contact Section Form*/}
<div className="row">
<div className="col-lg-8 mx-auto">
{/* To configure the contact form email address, go to mail/contact_me.php and update the email address in the PHP file on line 19.*/}
<form id="contactForm" name="sentMessage" noValidate="novalidate">
<div className="control-group">
<div className="form-group floating-label-form-group controls mb-0 pb-2">
<label>Name</label>
<input className="form-control" id="name" type="text" placeholder="Tên" required="required" data-validation-required-message="Please enter your name." />
<p className="help-block text-danger" />
</div>
</div>
<div className="control-group">
<div className="form-group floating-label-form-group controls mb-0 pb-2">
<label>Email Address</label>
<input className="form-control" id="email" type="email" placeholder="Địa chỉ email" required="required" data-validation-required-message="Please enter your email address." />
<p className="help-block text-danger" />
</div>
</div>
<div className="control-group">
<div className="form-group floating-label-form-group controls mb-0 pb-2">
<label>Phone Number</label>
<input className="form-control" id="phone" type="tel" placeholder="Số điện thoại liên hệ" required="required" data-validation-required-message="Please enter your phone number." />
<p className="help-block text-danger" />
</div>
</div>
<div className="control-group">
<div className="form-group floating-label-form-group controls mb-0 pb-2">
<label>Message</label>
<textarea className="form-control" id="message" rows={5} placeholder="Tin nhắn" required="required" data-validation-required-message="Please enter a message." defaultValue={""} />
<p className="help-block text-danger" />
</div>
</div>
<br />
<div id="success" />
<div className="form-group"><button className="btn btn-primary btn-xl" id="sendMessageButton" type="submit">Send</button></div>
</form>
</div>
</div>
</div>
</section>
);
}
}
export default SectionContact;
Bước 4 : Viết hàm App.js
Ở trong App.js, import các component và gọi trong hàm, đoạn code như sau :
App.js
import React from 'react';
import './App.css';
import Nav from './components/Nav.js'
import Header from "./components/Header";
import Section from "./components/Section";
import SectionAbout from "./components/SectionAbout";
import SectionContact from "./components/SectionContact";
import Footer from "./components/Footer";
function App() {
return (
<div className="App">
<Nav/>
<Header/>
<Section/>
<SectionAbout/>
<SectionContact/>
<Footer/>
</div>
);
}
export default App;
Vậy là đã xong một ứng dụng Reactjs từ bootstrap, các bạn chạy ứng dụng để xem thành quả :
npm start
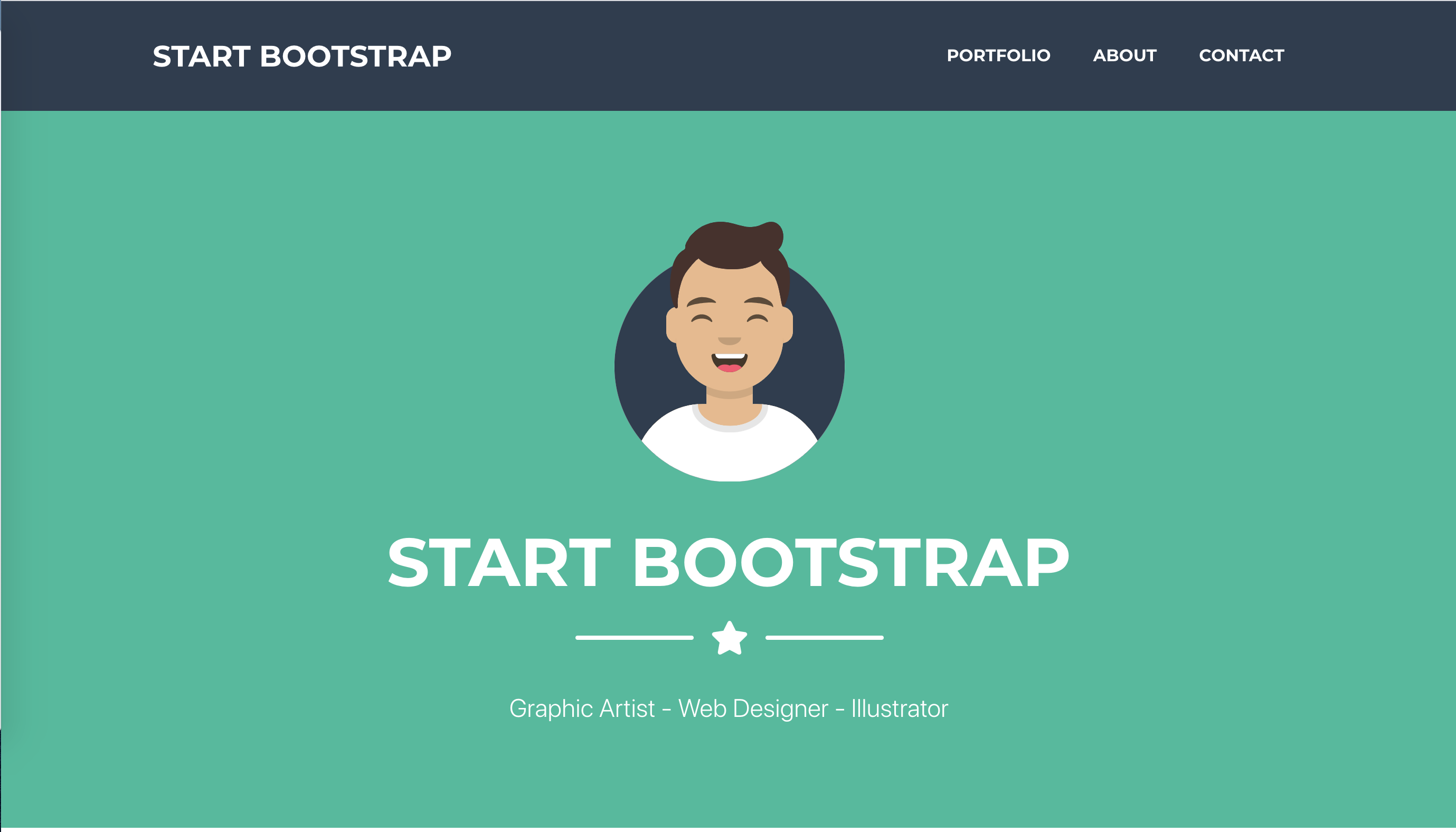
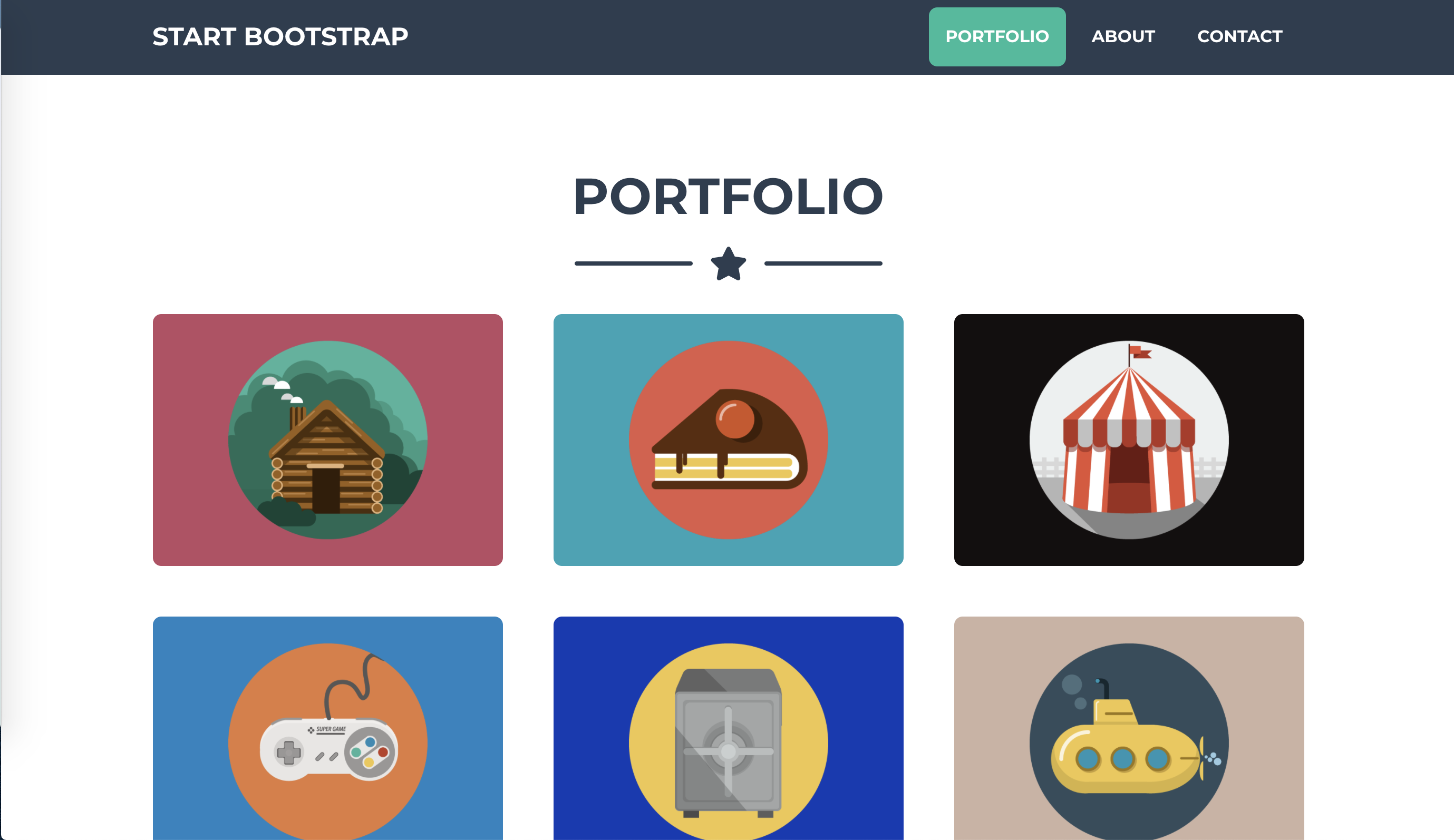
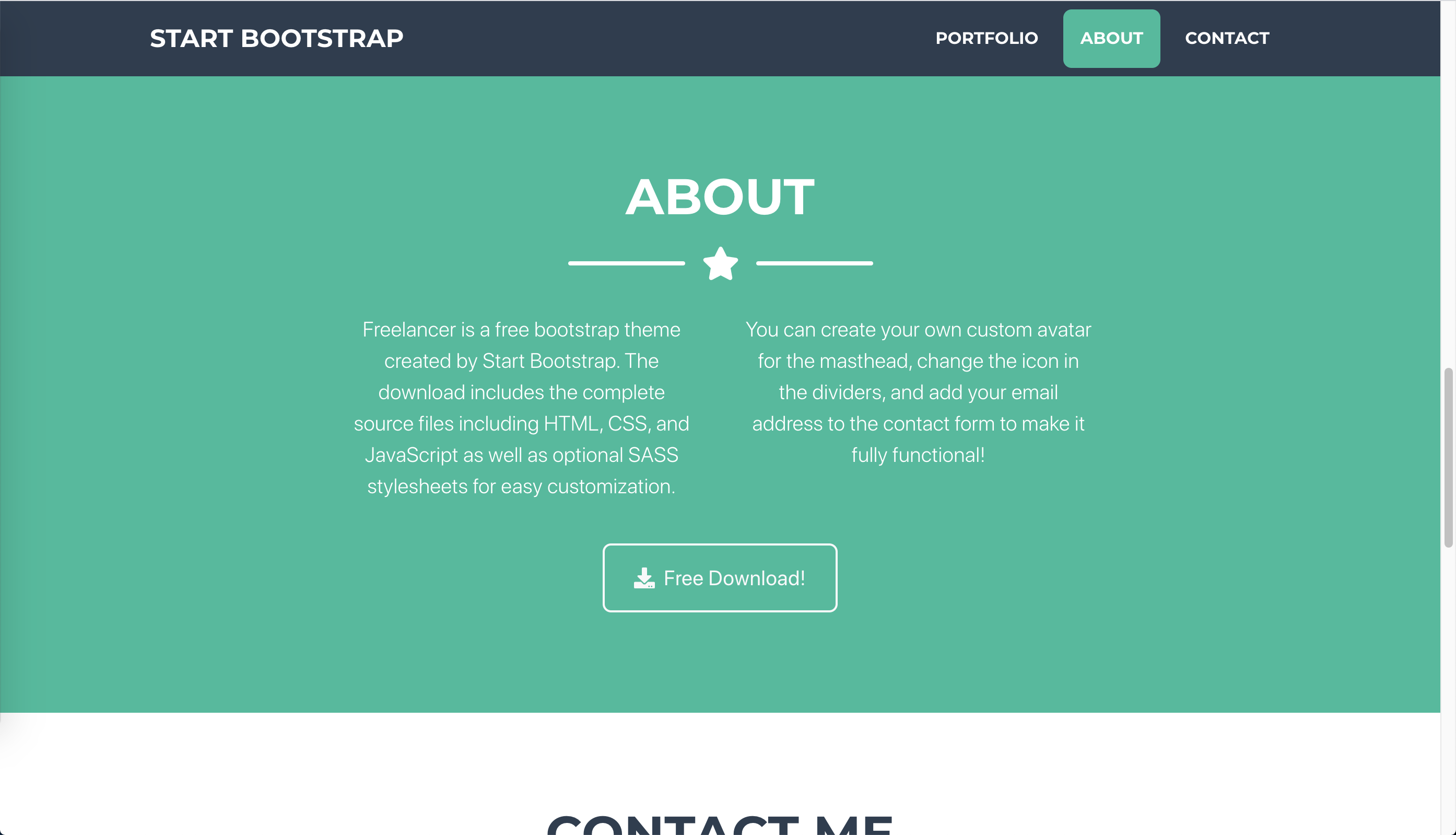
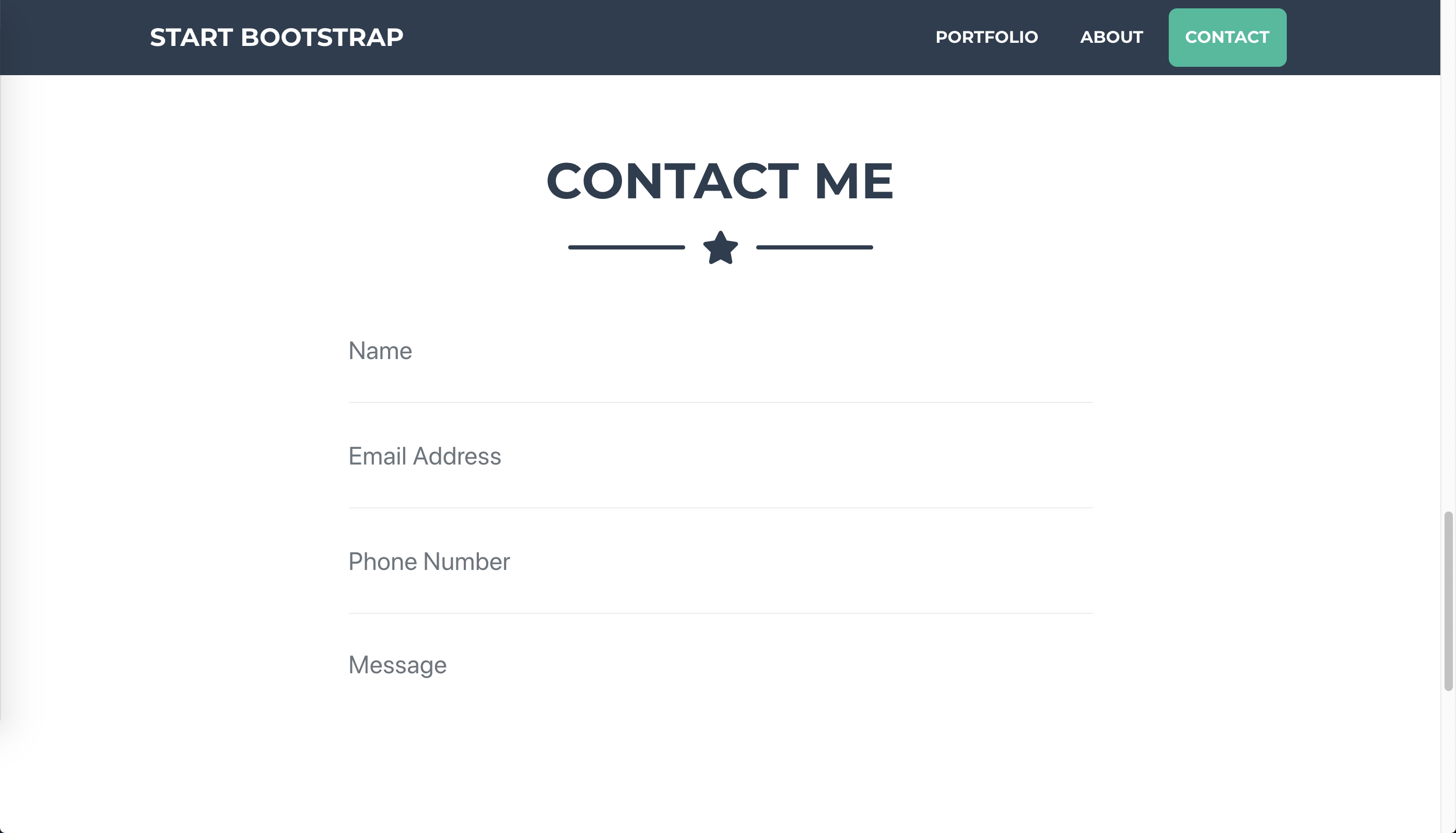
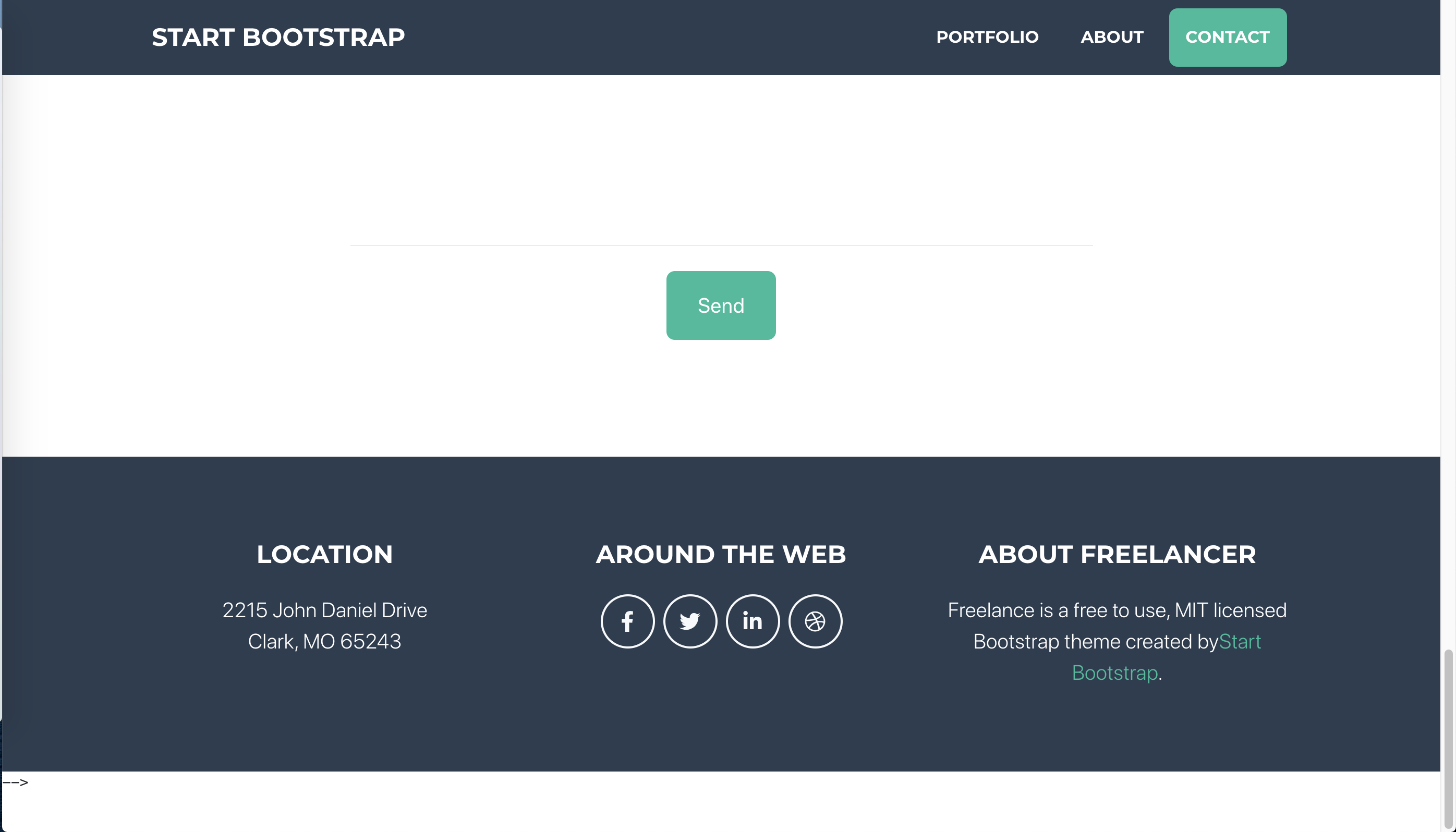
Cảm ơn các bạn đã theo dõi, hy vọng các bạn có thể thực hành nhiều ví dụ tương tự nắm vững hơn về Reactjs.
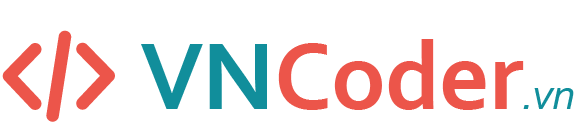
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
- Bài 1: ReactJS - Lời mở đầu
- Bài 2: ReactJS - Thiết lập môi trường
- Bài 3: ReactJS - JSX
- Bài 4: ReactJS - Components & State
- Bài 5: Props - Tổng quan
- Bài 6: Props - Validation
- Bài 7: ReactJJS - Component API
- Bài 8: ReactJS - Vòng đời của Component
- Bài 9: ReactJS - Forms
- Bài 10: ReactJS - Xử lý sự kiện
- Bài 11: ReactJS - Refs
- Bài 12: ReactJS - List và Keys
- Bài 13: ReactJS - Router
- Bài 14: ReactJS - Khái niệm Flux
- Bài 15: ReactJS - Cách sử dụng Flux
- Bài 16: ReactJS - Animation
- Bài 17: ReactJS - Higher Order Components
- Bài 18: ReactJS - Bản rút gọn(Quick guide - P1)
- Bài 19: ReactJS - Bản rút gọn(Quick guide - P2)
- Bài 20: ReactJS - Bản rút gọn(Quick guide - P3)
- Bài 21: ReactJS - Tạo ứng dụng reatjs từ bootstrap 4