- Bài 1: Thiết kế Database
- Bài 2: Xây dựng bố cục thư mục
- Bài 3: Tạo kết nối với CSDL
- Bài 4: Thiết kế giao diện cho trang Admin
- Bài 5: Làm chức năng đăng ký
- Bài 6: Làm chức năng đăng nhập cho người dùng
- Bài 7: Làm chức năng đăng nhập Admin
- Bài 8: Làm chức năng đăng xuất
- Bài 9: Thêm chuyên mục
- Bài 10: Làm chức năng sửa chuyên mục và hiển thị danh sách chuyên mục
- Bài 11: Làm chức năng thêm bài viết
- Bài 12: Làm chức năng hiển thị danh sách bài viết
- Bài 13: Làm chức năng sửa và xóa bài viết
Bài 10: Làm chức năng sửa chuyên mục và hiển thị danh sách chuyên mục - Xây dựng Website PHP theo MVC
Đăng bởi: Admin | Lượt xem: 4824 | Chuyên mục: PHP
1) Chuẩn bị
Đầu tiên chúng ta sẽ thiết kế giao diện danh sách chuyên mục và giao diện chỉnh sửa chuyên mục.
Tạo file View/admin/pages/catefory/list.php và chèn đoạn code sau:
<div class="content-wrapper" style="min-height: 1203.6px;">
<section class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1>Danh sách chuyên mục</h1>
</div>
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="#">Home</a></li>
<li class="breadcrumb-item active">Danh sách chuyên mục</li>
</ol>
</div>
</div>
</div>
</section>
<section>
<div class="col-12">
<div class="card">
<!-- /.card-header -->
<div class="card-body">
<div id="example2_wrapper" class="dataTables_wrapper dt-bootstrap4">
<div class="row">
<div class="col-sm-12 col-md-6"></div>
<div class="col-sm-12 col-md-6"></div>
</div>
<div class="row">
<div class="col-sm-12">
<table id="example2" class="table table-bordered table-hover dataTable" role="grid" aria-describedby="example2_info">
<thead>
<tr role="row">
<th class="sorting_asc" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-sort="ascending" aria-label="Rendering engine: activate to sort column descending">STT</th>
<th class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Browser: activate to sort column ascending">Tên chuyên mục</th>
<th style="text-align: center;" class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Platform(s): activate to sort column ascending">Sửa</th>
<th style="text-align: center;" class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Engine version: activate to sort column ascending">Xóa</th>
</tr>
</thead>
<tbody>
<tr role="row" class="odd">
<td class="sorting_1">1</td>
<td>Đời sống</td>
<td style="text-align: center;">
<span class="badge bg-primary">
<ion-icon name="create-outline"></ion-icon>
</span>
</td>
<td style="text-align: center;">
<span class="badge bg-danger">
<ion-icon name="trash-outline"></ion-icon>
</span>
</td>
</tr>
</tbody>
<tfoot>
<tr>
<th rowspan="1" colspan="1">STT</th>
<th rowspan="1" colspan="1">Tên chuyên mục</th>
<th rowspan="1" colspan="1">Sửa</th>
<th rowspan="1" colspan="1">Xóa</th>
</tr>
</tfoot>
</table>
</div>
</div>
</div>
</div>
<!-- /.card-body -->
</div>
</div>
</section>
</div>
Chạy chương trình theo đường dẫn sau: http://localhost/blog/View/admin/?controller=listCategory để xem kết quả:
Tiếp theo thiết kế giao diện sửa chuyên mục, tạo file View/admin/pages/catefory/edit.php và chèn đoạn code sau:
<div class="content-wrapper" style="min-height: 353px;">
<div class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1 class="m-0 text-dark">Sửa chuyên mục</h1>
</div><!-- /.col -->
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="#">Chuyên mục</a></li>
<li class="breadcrumb-item active">Admin</li>
</ol>
</div>
</div>
</div>
</div>
<section class="content">
<div class="container-fluid">
<form method="post">
<div class="card-body">
<div class="form-group">
<label>Tên chuyên mục</label>
<input type="text" name="name" class="form-control" placeholder="Tên chuyên mục">
</div>
<button type="submit" name="addCategory" class="btn btn-primary">Hoàn thành</button>
</div>
</form>
</div>
</section>
</div>
Chạy chương trình theo đường dẫn sau: http://localhost/blog/View/admin/?controller=editCategory để xem kết quả:
2) Làm chức năng hiển thị danh sách chuyên mục
Viết hàm categoryList lấy chuyên mục từ cơ sở dữ liệu trong file Model/admin/category.php:
public function categoryList()
{
$sql = "SELECT * FROM categories";
$result = $this->db->conn->query($sql);
$list = array();
while($data = $result->fetch_array()) {
$list[] = $data;
}
return $list;
}
File Model/admin/category.php hoàn chỉnh:
<?php
class CategoryModel extends Database{
protected $db;
public function __construct()
{
$this->db = new Database();
$this->db->connect();
}
public function addCategory($name, $slug)
{
$this->db->conn->real_escape_string($name);
$sql = "INSERT INTO categories (name, slug)
VALUES ('$name', '$slug')";
$this->db->conn->query($sql);
}
public function getCategory($categoryId)
{
$sql = "SELECT * FROM categories WHERE id = $categoryId";
$result = $this->db->conn->query($sql);
$data = $result->fetch_array();
return $data;
}
public function categoryList()
{
$sql = "SELECT * FROM categories";
$result = $this->db->conn->query($sql);
$list = array();
while($data = $result->fetch_array()) {
$list[] = $data;
}
return $list;
}
}
Bây giờ chúng ta xử lý logic bên Controller, tạo file Controller/admin/listCategory.php và chèn đoạn code sau:
<?php
class ListCategory {
public function __construct()
{
require_once('../../Model/admin/category.php');
$categoryModel = new CategoryModel();
$categories = $categoryModel->categoryList();
require('pages/category/list.php');
}
}
Hiển thị giữ liệu lấy được ra view, trong file View/admin/pages/catefory/list.php và chèn đoạn code sau:
<div class="content-wrapper" style="min-height: 1203.6px;">
<section class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1>Danh sách chuyên mục</h1>
</div>
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="#">Home</a></li>
<li class="breadcrumb-item active">Danh sách chuyên mục</li>
</ol>
</div>
</div>
</div>
</section>
<section>
<div class="col-12">
<div class="card">
<!-- /.card-header -->
<div class="card-body">
<div id="example2_wrapper" class="dataTables_wrapper dt-bootstrap4">
<div class="row">
<div class="col-sm-12 col-md-6"></div>
<div class="col-sm-12 col-md-6"></div>
</div>
<div class="row">
<div class="col-sm-12">
<?php
if (isset($_SESSION['thongbao'])) {?>
<div class="form-group alert alert-primary">
<?=$_SESSION['thongbao']?>
<?php unset($_SESSION['thongbao']); ?>
</div>
<?php }
?>
<table id="example2" class="table table-bordered table-hover dataTable" role="grid" aria-describedby="example2_info">
<thead>
<tr role="row">
<th class="sorting_asc" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-sort="ascending" aria-label="Rendering engine: activate to sort column descending">STT</th>
<th class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Browser: activate to sort column ascending">Tên chuyên mục</th>
<th style="text-align: center;" class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Platform(s): activate to sort column ascending">Sửa</th>
<th style="text-align: center;" class="sorting" tabindex="0" aria-controls="example2" rowspan="1" colspan="1" aria-label="Engine version: activate to sort column ascending">Xóa</th>
</tr>
</thead>
<tbody>
<?php
$stt = 0;
foreach ($categories as $category) {?>
<tr role="row" class="odd">
<td class="sorting_1"><?=++$stt?></td>
<td><?=$category['name']?></td>
<td style="text-align: center;">
<span class="badge bg-primary">
<a href="?controller=editCategory&categoryId=<?=$category['id']?>">
<ion-icon name="create-outline"></ion-icon>
</a>
</span>
</td>
<td style="text-align: center;">
<span class="badge bg-danger">
<a href="#">
<ion-icon name="trash-outline"></ion-icon>
</a>
</span>
</td>
</tr>
<?php }
?>
</tbody>
<tfoot>
<tr>
<th rowspan="1" colspan="1">STT</th>
<th rowspan="1" colspan="1">Tên chuyên mục</th>
<th rowspan="1" colspan="1">Sửa</th>
<th rowspan="1" colspan="1">Xóa</th>
</tr>
</tfoot>
</table>
</div>
</div>
</div>
</div>
<!-- /.card-body -->
</div>
</div>
</section>
</div>
Chạy chương trình theo đường dẫn sau: http://localhost/blog/View/admin/?controller=listCategory để xem kết quả
3) Làm chức năng sửa chuyên mục
Viết hàm getCategory lấy dữ liệu chuyên mục theo id trong file Model/admin/category.php:
public function getCategory($categoryId)
{
$sql = "SELECT * FROM categories WHERE id = $categoryId";
$result = $this->db->conn->query($sql);
$data = $result->fetch_array();
return $data;
}
Thêm hàm editCategory sửa chuyên mục trong file Model/admin/category.php:
public function editCategory($categoryId)
{
$sql = "UPDATE categories SET name = '$name' WHERE id = $categoryId";
return $this->db->conn->query($sql);
}
Bây giờ chúng ta xử lý logic bên Controller, tạo file Controller/admin/editCategory.php và chèn đoạn code sau:
<?php
class EditCategory {
public function __construct()
{
require_once('../../Model/admin/category.php');
$categoryModel = new CategoryModel();
$name = $slug = NULL;
if (isset($_GET['categoryId'])) {
$categoryId = $_GET['categoryId'];
$categoryOld = $categoryModel->getCategory($categoryId);
if (isset($_POST['editCategory'])) {
$name = $_POST['name'];
$slug = changeTitle($name);
$categoryModel->editCategory($name, $slug, $categoryId);
$_SESSION['thongbao'] = '* Thêm thành công';
header('Location: ?controller=listCategory');
}
require('pages/category/edit.php');
}
}
}
Hiển thị tên chuyên mục cũ muốn sửa trong file View/admin/pages/category/edit:
<div class="content-wrapper" style="min-height: 353px;">
<div class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1 class="m-0 text-dark">Sửa chuyên mục</h1>
</div><!-- /.col -->
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="#">Chuyên mục</a></li>
<li class="breadcrumb-item active">Admin</li>
</ol>
</div>
</div>
</div>
</div>
<section class="content">
<div class="container-fluid">
<form method="post">
<div class="card-body">
<div class="form-group">
<label>Tên chuyên mục</label>
<input type="text" value="<?=$categoryOld['name']?>" name="name" class="form-control" placeholder="Tên chuyên mục">
</div>
<button type="submit" name="editCategory" class="btn btn-primary">Hoàn thành</button>
</div>
</form>
</div>
</section>
</div>
Hiển thị thông báo sau khi sửa thành công, chèn đoạn code sau vào file View/admin/pages/category/list:
<?php
if (isset($_SESSION['thongbao'])) {?>
<div class="form-group alert alert-primary">
<?=$_SESSION['thongbao']?>
<?php unset($_SESSION['thongbao']); ?>
</div>
<?php }
?>
Chạy chương trình theo đường dẫn sau: http://localhost/blog/View/admin/?controller=editCategory&categoryId=$category để chỉnh sửa chuyên mục. Biến $category sẽ là giá trị cột id trong bảng categories.
Như vậy mình đã hướng dẫn các bạn hoàn thành chức năng hiển thị danh sách chuyên mục và sửa chuyên mục. Nếu có gì không hiểu hãy comment bên dưới để được giải đáp. Chúc các bạn thành công!.
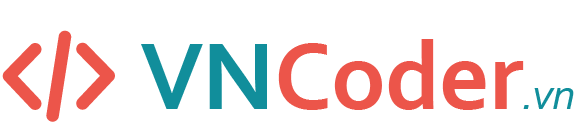
Theo dõi VnCoder trên Facebook, để cập nhật những bài viết, tin tức và khoá học mới nhất!
- Bài 1: Thiết kế Database
- Bài 2: Xây dựng bố cục thư mục
- Bài 3: Tạo kết nối với CSDL
- Bài 4: Thiết kế giao diện cho trang Admin
- Bài 5: Làm chức năng đăng ký
- Bài 6: Làm chức năng đăng nhập cho người dùng
- Bài 7: Làm chức năng đăng nhập Admin
- Bài 8: Làm chức năng đăng xuất
- Bài 9: Thêm chuyên mục
- Bài 10: Làm chức năng sửa chuyên mục và hiển thị danh sách chuyên mục
- Bài 11: Làm chức năng thêm bài viết
- Bài 12: Làm chức năng hiển thị danh sách bài viết
- Bài 13: Làm chức năng sửa và xóa bài viết